HTML, CSS, and JavaScript form the foundational pillars of modern web development. Whether you’re a novice developer aiming to break into the tech industry or an experienced coder seeking to refine your skills, preparing for interviews is crucial. These three technologies enable the creation of interactive, visually appealing, and responsive web applications. In interviews, questions typically span a range of topics, testing your understanding of basic syntax, advanced functionalities, and the ability to solve real-world problems.
In this article, we’ll cover the top 37 HTML, CSS, and JavaScript interview questions, providing concise answers with explanations to ensure you’re fully prepared for your next technical interview.
Top 37 HTML, CSS, and JavaScript Interview Questions
1. What is HTML, and why is it important in web development?
HTML (Hypertext Markup Language) is the standard language used to structure web pages and their content. It uses tags to organize text, images, links, and other elements. Without HTML, web browsers wouldn’t understand how to display the elements correctly on a web page.
Explanation:
HTML acts as the backbone of any web page, structuring the information so that browsers can render it properly.
2. What are the different types of CSS and where can it be applied?
CSS can be applied in three ways: inline (within HTML tags), internal (within the <style>
tag in the head), and external (in a separate .css
file). Each has its own use case based on scope and reusability.
Explanation:
External CSS files are commonly used for larger projects, while inline and internal are best for quick fixes or small projects.
3. What is the difference between a class and an ID in CSS?
A class can be applied to multiple elements, while an ID is unique to a single element on a page. Classes use a dot (.
) in the selector, and IDs use a hash (#
).
Explanation:
Classes are reusable, making them more flexible, while IDs should be reserved for unique elements like headers.
4. What is the box model in CSS?
The box model consists of four areas: content, padding, border, and margin. It determines how elements are sized and spaced on a webpage.
Explanation:
Understanding the box model is essential for creating layouts that align elements accurately across devices.
5. What is the difference between display: block
and display: inline
in CSS?
display: block
makes an element take up the full width of its container, while display: inline
allows elements to sit next to each other horizontally without breaking the flow of content.
Explanation:
Block elements like <div>
are useful for creating sections, while inline elements are ideal for text or small images.
Build your resume in just 5 minutes with AI.
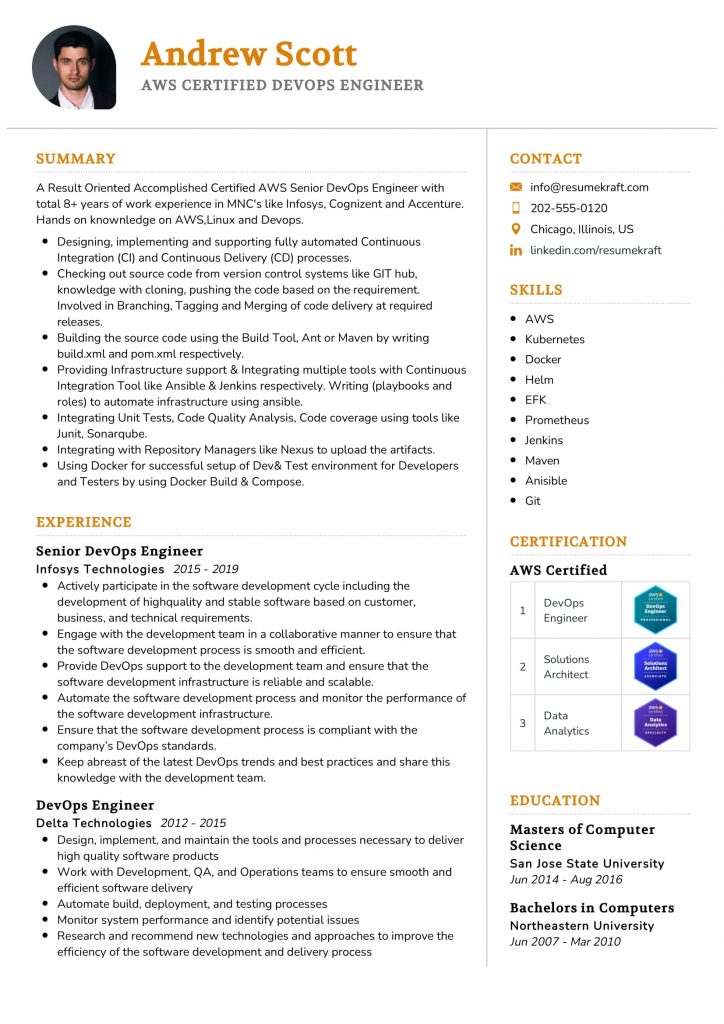
6. What is Flexbox in CSS, and how is it used?
Flexbox is a CSS layout model that allows elements to align and distribute space within a container efficiently. It is particularly useful for building responsive designs without relying on floats or positioning.
Explanation:
Flexbox simplifies vertical and horizontal alignment tasks, making it easier to build complex layouts with minimal code.
7. What is the purpose of the z-index
property in CSS?
The z-index
property controls the stacking order of elements. Elements with a higher z-index
appear above those with a lower z-index
.
Explanation:
This property is particularly important for overlapping elements, ensuring that the most important ones are visible.
8. What are media queries in CSS?
Media queries allow developers to apply different styles depending on the screen size or device type. They are essential for building responsive websites.
Explanation:
Media queries help create websites that look good on mobile, tablet, and desktop devices by adjusting layouts and styles accordingly.
9. What is the Document Object Model (DOM)?
The DOM is a programming interface that represents the structure of a web page. JavaScript can interact with the DOM to manipulate elements, styles, and attributes dynamically.
Explanation:
By using the DOM, developers can change content and styles on the fly, enhancing interactivity and user experience.
10. What are JavaScript variables, and how are they declared?
Variables in JavaScript store data values and can be declared using var
, let
, or const
. Each has its own scope and behavior.
Explanation:
Using let
and const
is preferable over var
because they provide block-level scope, reducing the risk of accidental redeclarations.
11. What is the difference between let
and const
in JavaScript?
let
allows you to reassign a variable, while const
prevents reassignment after the initial value is set.
Explanation:
Using const
ensures that values like configurations or constants don’t get changed accidentally, improving code reliability.
12. What is the difference between synchronous and asynchronous programming in JavaScript?
Synchronous programming executes code line by line, blocking further execution until the current task is complete. Asynchronous programming, on the other hand, allows tasks to run in the background without blocking the main thread.
Explanation:
JavaScript uses async programming to handle time-consuming tasks like API requests without freezing the user interface.
13. How does the this
keyword work in JavaScript?
The this
keyword refers to the current object in context. Its value changes depending on how a function is called.
Explanation:
Understanding this
is crucial when working with object-oriented JavaScript, as it affects how methods and properties are accessed.
14. What is event delegation in JavaScript?
Event delegation is a technique where a single event listener is attached to a parent element to manage events from its child elements. This reduces the number of event listeners required.
Explanation:
Event delegation improves performance by reducing the number of event handlers, especially in dynamically created elements.
15. What are JavaScript promises, and how do they work?
A promise represents the eventual completion or failure of an asynchronous operation. It allows you to chain operations using .then()
and .catch()
.
Explanation:
Promises simplify handling asynchronous code by avoiding deeply nested callbacks, commonly known as “callback hell.”
16. What is the difference between ==
and ===
in JavaScript?
==
checks for value equality, performing type coercion if necessary, while ===
checks for both value and type equality.
Explanation:
Using ===
is generally recommended because it avoids unexpected type conversions that may lead to bugs.
17. How can you prevent default behavior in JavaScript events?
You can use the event.preventDefault()
method to stop the default action of an event, such as preventing a form from submitting.
Explanation:
Preventing default behavior is useful for custom form validation or handling special cases like single-page applications.
18. What are closures in JavaScript?
A closure is a function that remembers its lexical scope even after it has been executed. This allows for private variables and functions within JavaScript.
Explanation:
Closures are powerful for data encapsulation, enabling the creation of private variables that aren’t accessible from outside the function.
19. How does the addEventListener
method work in JavaScript?
addEventListener
attaches an event handler to an element without overwriting existing handlers. It takes three parameters: the event type, the callback function, and an optional useCapture flag.
Explanation:
Using addEventListener
is the modern way to add event listeners and allows for multiple handlers on the same event.
20. What is the difference between null
and undefined
in JavaScript?
undefined
means a variable has been declared but not assigned a value, while null
is an assignment value that represents “no value.”
Explanation:
Distinguishing between null
and undefined
is crucial in JavaScript to avoid type errors during comparisons and operations.
21. How does setTimeout
work in JavaScript?
setTimeout
schedules a function to execute after a specified amount of time, measured in milliseconds.
Explanation:
It is a basic function for handling asynchronous tasks, such as animations, without freezing the main execution thread.
22. What are arrow functions in JavaScript, and how do they differ from regular functions?
Arrow functions are a concise way to write functions in JavaScript. Unlike regular functions, they do not have their own this
binding.
Explanation:
Arrow functions are often used in callbacks because they retain the this
value from their enclosing context, simplifying code.
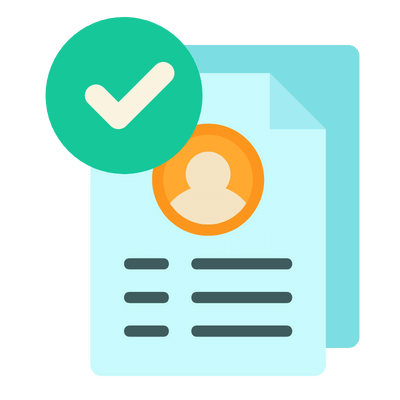
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
23. What are CSS animations, and how do they work?
CSS animations allow for the gradual change of CSS properties over time. You can define keyframes for each stage of the animation, along with its duration and timing function.
Explanation:
Animations enhance user experience by adding dynamic visual effects without relying on JavaScript for transitions.
24. What is the difference between margin
and padding
in CSS?
margin
controls the space outside an element, while padding
controls the space inside an element’s border.
Explanation:
Understanding the difference is key to building well-structured layouts where elements are properly spaced from each other.
25. How do you create a responsive web page?
You create a responsive web page by using flexible grids, media queries, and flexible images. The page should adjust to different screen sizes and resolutions.
Explanation:
Responsive design ensures that a website looks and performs well across a variety of devices, including smartphones and tablets.
26. What is the purpose of the async
and defer
attributes in HTML?
The async
attribute loads
scripts asynchronously, while defer
ensures that the script is executed after the HTML document has been parsed.
Explanation:
Both attributes help in optimizing page load times by controlling when and how scripts are executed.
27. How does the localStorage
API work in JavaScript?
The localStorage
API provides a way to store key-value pairs in a web browser with no expiration time, persisting across page reloads and browser sessions.
Explanation:
It is commonly used for saving user preferences, shopping cart items, or other stateful data in web applications.
28. What is AJAX, and how does it work?
AJAX (Asynchronous JavaScript and XML) allows web applications to update parts of a web page without reloading the entire page. It works by sending HTTP requests in the background and handling responses dynamically.
Explanation:
AJAX improves user experience by making web applications faster and more interactive through asynchronous data fetching.
29. How do you include JavaScript in an HTML document?
You can include JavaScript in an HTML document using the <script>
tag. Scripts can be placed in the <head>
, <body>
, or externally linked using the src
attribute.
Explanation:
Including JavaScript allows you to add interactivity and dynamic functionality to web pages.
30. What is JSON, and how is it used in JavaScript?
JSON (JavaScript Object Notation) is a lightweight data format used for exchanging data between a server and a client. It is easy to read and write for humans and machines alike.
Explanation:
JSON has become the standard format for sending data via APIs due to its simplicity and compatibility with most programming languages.
31. What is the difference between inline, block, and inline-block elements in HTML/CSS?
Inline elements only take up as much space as necessary and don’t break the line (e.g., <span>
). Block elements take up the full width of their parent container (e.g., <div>
). Inline-block combines the characteristics of both.
Explanation:
Choosing the right display property affects layout and alignment, helping you achieve the desired design.
32. What is the difference between window.onload
and DOMContentLoaded
in JavaScript?
window.onload
fires after the entire page, including stylesheets and images, has loaded. DOMContentLoaded
fires as soon as the DOM is fully loaded, without waiting for external resources.
Explanation:
Using DOMContentLoaded
ensures your scripts execute as soon as the HTML is ready, speeding up page interaction.
33. What is hoisting in JavaScript?
Hoisting is JavaScript’s default behavior of moving declarations to the top of the current scope before code execution. However, only variable declarations are hoisted, not initializations.
Explanation:
Understanding hoisting helps avoid issues where variables or functions seem to be used before they’re declared.
34. How can you make a form accessible in HTML?
You can make a form accessible by using semantic HTML elements like <label>
and providing descriptive text for form inputs, ensuring screen readers can read the form fields correctly.
Explanation:
Accessibility is crucial for ensuring that users with disabilities can interact with web applications effectively.
35. What are pseudo-classes in CSS, and how are they used?
Pseudo-classes are used to define the state of an element. For example, :hover
applies a style when a user hovers over an element, and :nth-child
applies styles based on the position of an element among its siblings.
Explanation:
Pseudo-classes allow for interactive styling without JavaScript, improving both aesthetics and user experience.
36. How does the CSS float
property work?
The float
property moves elements to the left or right within their container, allowing text and inline elements to wrap around them. However, it can cause layout issues if not cleared properly.
Explanation:
Floats were once widely used for layouts, but now, Flexbox and Grid are preferred for their simplicity and reliability.
37. What is the reduce()
method in JavaScript?
The reduce()
method in JavaScript executes a reducer function on each element of an array, resulting in a single output value. It is useful for tasks like summing up values or transforming data into a new structure.
Explanation:reduce()
is a powerful method for data processing and aggregation in functional programming, improving code clarity and performance.
Conclusion
Mastering HTML, CSS, and JavaScript is essential for web developers, as these technologies are the backbone of the web. Interview questions often test both fundamental and advanced knowledge, so thorough preparation is crucial. Whether you’re applying for a front-end developer role or a full-stack position, understanding these key concepts will boost your confidence and performance during interviews.
As you prepare, don’t forget to practice building projects, such as creating a resume builder, or exploring free resume templates and resume examples to enhance your web development skills.
Recommended Reading: