Tesla is one of the most innovative companies, constantly pushing the boundaries in technology. If you’re applying for a front-end position at Tesla, you can expect your interview to be rigorous, especially if it involves React. Tesla values problem-solving, efficient coding, and a deep understanding of React principles. In this article, we’ll explore the most common React interview questions asked at Tesla, along with answers and explanations to help you prepare.
Top 37 Tesla React Interview Questions
1. What is React, and why is it used?
React is a JavaScript library developed by Facebook for building user interfaces, especially for single-page applications. It allows developers to create reusable UI components, enabling efficient and scalable application development.
Explanation:
React is preferred because of its virtual DOM implementation and component-based architecture, which simplifies UI updates and improves performance.
2. What are the main features of React?
React focuses on component-based architecture, declarative UI, unidirectional data flow, and JSX syntax for combining JavaScript and HTML.
Explanation:
React’s main features make it powerful for building dynamic user interfaces and provide a clear structure for managing large-scale applications.
3. What is the virtual DOM?
The virtual DOM is a lightweight representation of the real DOM. React keeps a copy of the DOM in memory and synchronizes it with the real DOM using a process called reconciliation.
Explanation:
Using the virtual DOM, React minimizes the number of direct manipulations with the real DOM, making updates faster and more efficient.
4. What are components in React?
Components are the building blocks of a React application. They encapsulate HTML, CSS, and JavaScript to create reusable UI elements.
Explanation:
React components allow developers to break the UI into smaller, manageable pieces, making the code more modular and maintainable.
5. Explain the difference between functional and class components.
Functional components are stateless and simpler to write, while class components are stateful and provide more features like lifecycle methods.
Explanation:
Functional components have gained popularity due to the introduction of React Hooks, which allow them to handle state and other side effects.
6. What is JSX in React?
JSX stands for JavaScript XML. It allows you to write HTML-like syntax within JavaScript code. JSX is then compiled into React.createElement calls.
Explanation:
JSX makes React code more readable and easier to write by merging the structure of HTML with the power of JavaScript.
Build your resume in just 5 minutes with AI.
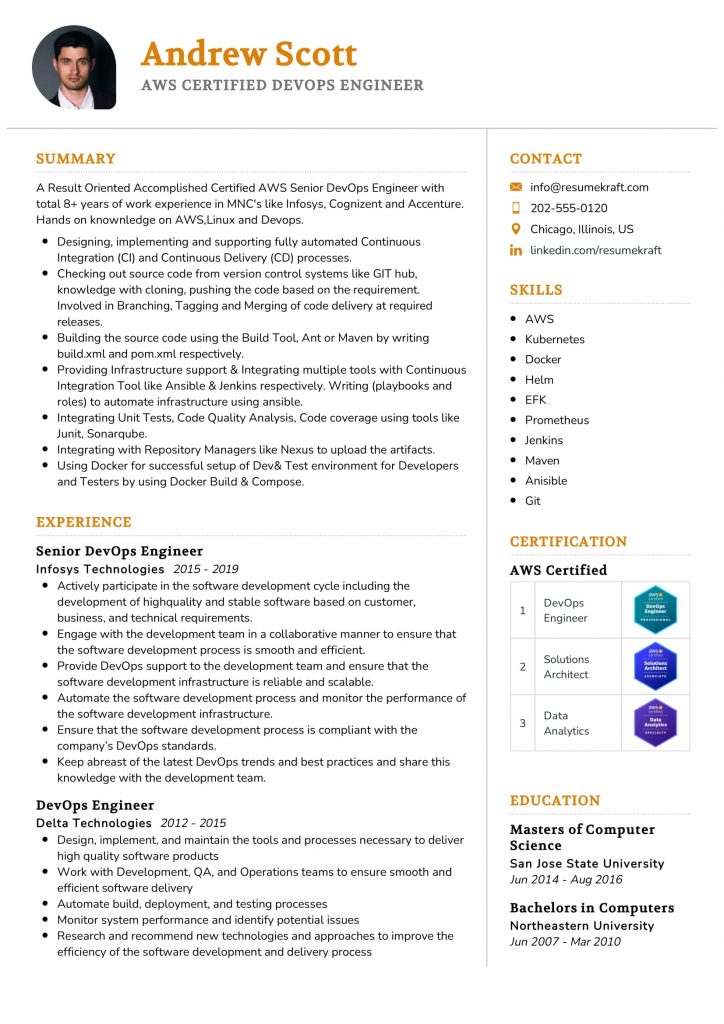
7. How does React handle events?
React normalizes events across different browsers using a system known as synthetic events, which ensures consistent behavior regardless of the environment.
Explanation:
This normalization helps developers avoid cross-browser issues and ensures the code runs smoothly across different platforms.
8. What is a state in React?
State is a JavaScript object that holds information that influences the output of a component. It is used to keep track of changes in data over time.
Explanation:
State management allows React components to update dynamically, reflecting changes in the UI without reloading the page.
9. What is a prop in React?
Props (short for properties) are read-only inputs passed from one component to another. They help components communicate with each other.
Explanation:
Props provide a way to pass data between components, making it possible to create dynamic and flexible UI elements.
10. What is the difference between state and props?
State is internal to a component and can change over time, while props are external inputs passed to a component and are immutable.
Explanation:
Props are for passing data, while state is for managing component-specific data that may change over time.
11. How do you handle conditional rendering in React?
Conditional rendering in React can be handled using JavaScript expressions such as ternary operators, if-else statements, or logical && operators within JSX.
Explanation:
Conditional rendering allows you to render different UI elements or components based on specific conditions in your application.
12. What is the useEffect hook?
The useEffect
hook allows you to perform side effects in functional components, such as fetching data, subscribing to services, or manually updating the DOM.
Explanation:
It replaces lifecycle methods like componentDidMount and componentDidUpdate in class components, providing a more intuitive approach to handling side effects.
13. What is the useState hook?
The useState
hook is used to add state to functional components. It returns a stateful value and a function to update it.
Explanation:
The useState
hook makes it possible for functional components to manage state, making them more versatile and reducing the need for class components.
14. What are React fragments?
React Fragments allow you to group multiple elements without adding extra nodes to the DOM, preventing unnecessary DOM updates.
Explanation:
Fragments improve performance by preventing the addition of unnecessary elements, keeping the DOM cleaner and more efficient.
15. What is React Router, and why is it used?
React Router is a library for routing in React applications. It enables navigation between different components without reloading the page.
Explanation:
React Router is essential for building single-page applications (SPAs) where different views need to be rendered based on user actions or URLs.
16. How does React Router handle nested routes?
React Router uses a component-based approach to define nested routes. Each route can render a component and contain child routes that render other components.
Explanation:
Nested routes help structure the navigation in complex applications, allowing for multi-level navigation flows.
17. What is the context API in React?
The Context API is a way to pass data through the component tree without having to pass props manually at every level.
Explanation:
It solves the problem of “prop drilling,” where data has to be passed through multiple levels of components unnecessarily.
18. What are React keys, and why are they important?
Keys are unique identifiers used by React to keep track of elements in lists, enabling efficient updates to the DOM when the list changes.
Explanation:
React uses keys to optimize rendering performance by identifying which elements have changed, been added, or removed.
19. What is a controlled component in React?
A controlled component is a form element whose value is controlled by React state, meaning that the component’s state drives the form’s behavior.
Explanation:
Controlled components provide a consistent way to manage form input by synchronizing the form’s state with the application state.
20. What is an uncontrolled component in React?
An uncontrolled component is a form element that manages its own state internally, rather than relying on React state to control its behavior.
Explanation:
Uncontrolled components allow form elements to operate independently, but they offer less control over input management.
21. What is prop drilling in React?
Prop drilling refers to passing props through multiple layers of components to reach a deeply nested component, which can make the code harder to manage.
Explanation:
Prop drilling can lead to complex and difficult-to-maintain code, which is why patterns like the Context API or state management libraries are often used.
22. What is React.memo?
React.memo
is a higher-order component that prevents unnecessary re-rendering of functional components by memoizing their output.
Explanation:
It improves performance by preventing components from re-rendering if their props haven’t changed.
23. What is lazy loading in React?
Lazy loading is a technique for loading components only when they are needed, improving the performance of large applications by splitting the bundle.
Explanation:
Lazy loading reduces the initial load time of an application, as it only loads components when they are required.
24. What is code splitting in React?
Code splitting is a technique that splits your code into smaller chunks, which are loaded on demand, improving the performance of React applications.
Explanation:
Code splitting allows your application to load faster by reducing the size of the initial JavaScript bundle.
25. How do you optimize a React application?
Optimizing a React application involves using techniques like code splitting, lazy loading, memoization, and reducing the number of re-renders through efficient state management.
Explanation:
Optimization techniques help improve the performance and user experience of React applications, especially in large-scale projects.
26. What is a higher-order component (HOC)?
A higher-order component is a function that takes a component and returns a new component, enhancing the functionality of the original component.
Explanation:
HOCs provide a way to reuse logic across multiple components, promoting code reusability and separation of concerns.
27. What is reconciliation in React?
Reconciliation is the process React uses to update the DOM by comparing the virtual DOM with the real DOM and making only the necessary changes.
Explanation:
Reconciliation ensures that the UI remains efficient and up-to-date without requiring a full re-render of the entire DOM.
28. What are pure components?
Pure components in React are components that only re-render when their props or state change. They perform shallow comparisons of props and state.
Explanation:
Pure components improve performance by avoiding unnecessary re-renders, particularly in large applications.
29. What is the difference between forEach and map in React?
forEach
is used for iterating over an array without returning a new array, while map
returns a new array with the results of calling a function on every element.
Explanation:
*Using map
in React is more common
because it allows you to generate new arrays of JSX elements, while forEach
is purely for side effects.*
30. How do you handle form validation in React?
Form validation in React can be handled by managing the form’s state and performing checks within the component or by using libraries like Formik or Yup for more complex validations.
Explanation:
Handling validation ensures that user input is properly managed and error messages are displayed appropriately.
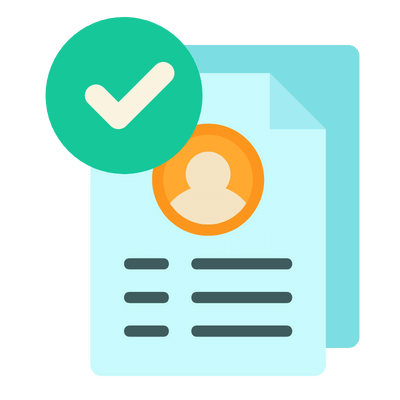
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
31. How does React handle performance optimization?
React optimizes performance using techniques like virtual DOM, memoization (React.memo
), and hooks like useMemo
and useCallback
to avoid unnecessary re-renders.
Explanation:
React’s built-in optimization features help maintain a smooth user experience by reducing the amount of work required during updates.
32. What is the difference between synchronous and asynchronous rendering in React?
Synchronous rendering updates the UI immediately, while asynchronous rendering allows React to prioritize updates, ensuring that important UI updates are not blocked.
Explanation:
Asynchronous rendering provides better performance for complex applications, especially when dealing with heavy computations or large data sets.
33. How does React handle accessibility?
React supports accessibility by providing standard web practices like semantic HTML and WAI-ARIA standards. It also offers tools like React’s aria-
attributes.
Explanation:
Ensuring accessibility makes applications usable for a wider audience, including users with disabilities.
34. What is the role of hooks in React?
Hooks allow functional components to use features like state and lifecycle methods, making it easier to manage side effects and logic in functional components.
Explanation:
Hooks simplify state management in functional components, reducing the need for class components.
35. How does React handle SEO?
React applications, especially single-page apps, may face SEO challenges due to client-side rendering. Solutions like server-side rendering (SSR) with Next.js or pre-rendering can improve SEO.
Explanation:
Optimizing for SEO in React applications involves ensuring that search engines can index the content properly, even if it’s rendered dynamically.
36. What are some common performance issues in React applications?
Common performance issues include excessive re-renders, large bundles, inefficient state management, and failing to memoize functions or components.
Explanation:
Addressing these issues can significantly improve the speed and responsiveness of a React application.
37. What is the role of Webpack in React?
Webpack is a module bundler used in React projects to bundle JavaScript files, CSS, images, and other assets. It helps optimize the application for production by minimizing the bundle size.
Explanation:
Webpack plays a crucial role in improving the performance of React applications by reducing the amount of code that needs to be loaded.
Conclusion
Preparing for a React interview at Tesla can be challenging, but with a thorough understanding of these concepts, you’ll be well-equipped to tackle any technical questions. Remember to focus on key React principles such as component-based architecture, efficient state management, and performance optimization. Whether you’re applying for Tesla or any other leading tech company, mastering React’s core features will put you ahead of the competition.
For further preparation, make sure your resume stands out by using a resume builder that highlights your technical skills effectively. Explore free resume templates and resume examples to get ideas for how to present your experience in the best possible light.
Good luck with your interview preparation, and may you ace your Tesla React interview!
Recommended Reading: