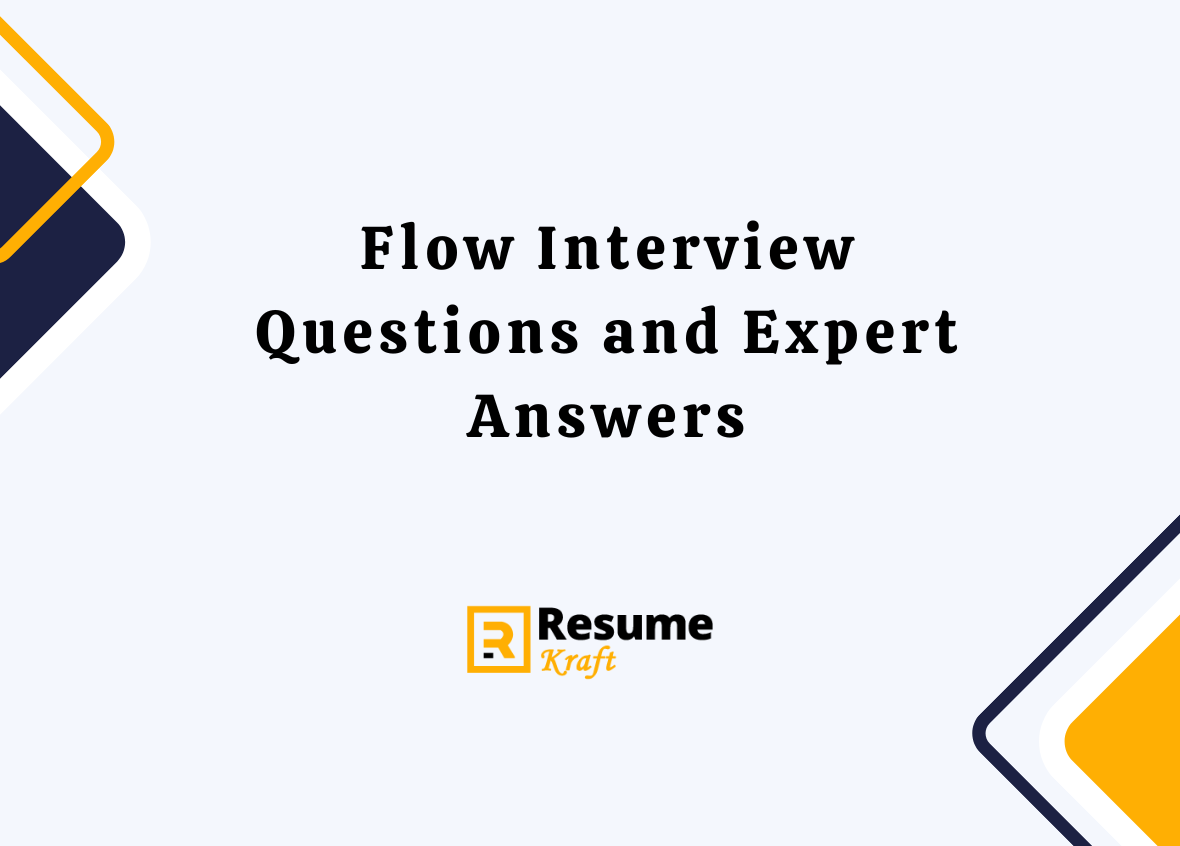
Flow is a vital concept in computer programming and software development. It refers to the smooth progression and coordination of data and processes within a system, ensuring that operations execute in the desired sequence and meet performance requirements. In a flow-based interview, candidates are tested on their understanding of the control flow, event-driven architecture, process management, and asynchronous programming. The interviewer may also assess problem-solving abilities, logic, and efficiency. In this article, we will explore 27 common flow interview questions, along with brief explanations to help you understand the thought process behind each.
Top 27 Flow Interview Questions and Answers
1. What is control flow in programming?
Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated in a program. Typically, a program starts from the first line of code and proceeds sequentially unless influenced by control structures such as loops, conditionals, and function calls.
Explanation: Control flow is foundational to programming logic as it dictates how a program runs. Without control flow mechanisms, programs would lack structure, making complex tasks nearly impossible to achieve.
2. Can you explain the concept of flow in asynchronous programming?
In asynchronous programming, flow refers to the management of tasks that run independently of the main program flow. These tasks are executed in the background, and the program continues without waiting for them to complete. Promises, callbacks, and async/await are commonly used to manage this flow.
Explanation: Asynchronous flow allows programs to remain responsive and efficient by handling long-running tasks without blocking the main thread.
3. What is data flow in a system, and why is it important?
Data flow in a system represents the movement of data from one part of the system to another. Understanding how data flows through different modules, services, or components is crucial for designing scalable and maintainable systems.
Explanation: Managing data flow properly ensures that the system processes information efficiently, reduces errors, and maintains synchronization between components.
4. How does control flow differ from data flow?
Control flow deals with the order in which operations are executed, while data flow focuses on how data moves through the system. Control flow controls logic execution, while data flow ensures the correct transmission of data between different parts of the program.
Explanation: These two concepts are complementary; control flow structures the logic, and data flow ensures that the information needed to execute that logic is available at the right time.
Build your resume in just 5 minutes with AI.
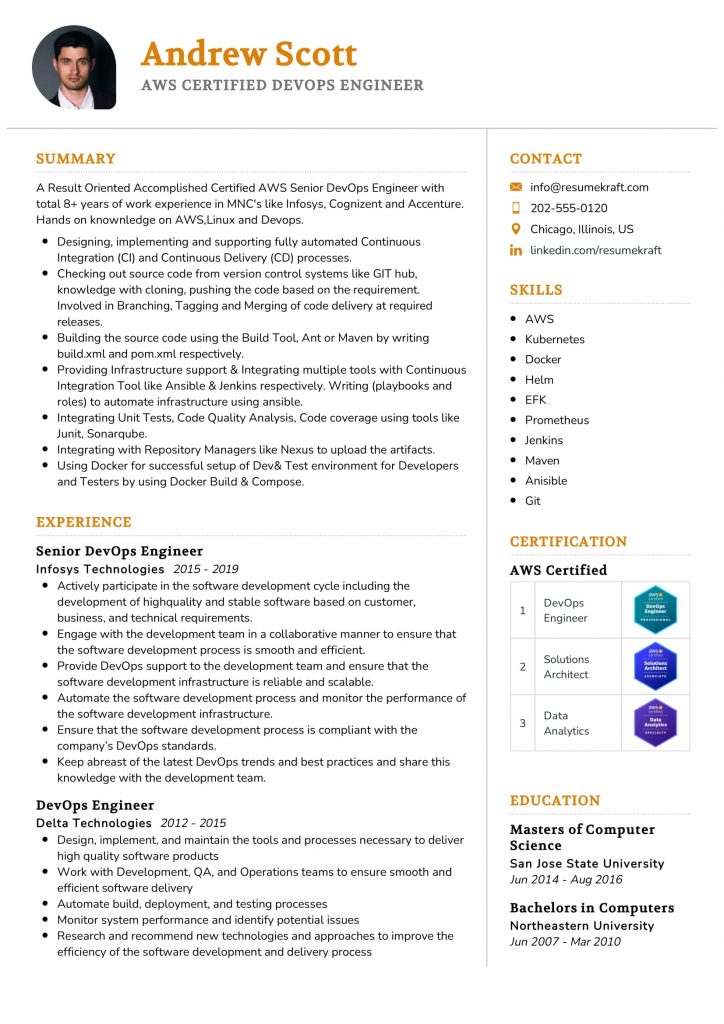
5. What are conditional statements in control flow?
Conditional statements such as if
, else
, and switch
allow programs to make decisions based on specific conditions. Depending on whether a condition is true or false, the program can follow different branches of execution.
Explanation: Conditional statements are essential for introducing decision-making into a program, enabling dynamic behavior and flexibility.
6. What is the purpose of loops in flow control?
Loops allow a block of code to be executed repeatedly based on a condition. Common loop types include for
, while
, and do-while
loops. They help in automating repetitive tasks within a program.
Explanation: Loops are useful for iterating over collections, processing items multiple times, or repeatedly checking conditions.
7. Explain the difference between synchronous and asynchronous flow.
In synchronous flow, operations are executed one after another, with each step waiting for the previous one to complete. Asynchronous flow allows multiple operations to happen concurrently without waiting for others to finish.
Explanation: Synchronous flow is simpler but can be inefficient for long-running tasks. Asynchronous flow improves performance but requires more complex handling of concurrency.
8. How do callbacks help manage asynchronous flow?
Callbacks are functions passed as arguments to other functions, which are executed once an asynchronous operation is complete. They help ensure that tasks are handled after the previous operation finishes.
Explanation: Callbacks manage the flow of asynchronous operations, but they can lead to callback hell, where the code becomes deeply nested and hard to read.
9. What are promises in JavaScript, and how do they improve flow control?
Promises represent an eventual completion (or failure) of an asynchronous operation. Unlike callbacks, promises chain operations and handle success and failure more elegantly, making asynchronous flow easier to manage.
Explanation: Promises reduce the complexity of nested callbacks and make the code more readable and maintainable.
10. How does the async/await
syntax improve flow control in asynchronous programming?
The async/await
syntax simplifies working with promises by allowing developers to write asynchronous code that looks synchronous. It pauses the execution until the awaited promise is resolved, improving readability.
Explanation: Async/await
improves the flow of asynchronous operations by flattening the callback chains and making the code easier to follow.
11. What is event-driven flow?
Event-driven flow refers to the architecture where the flow of the program is determined by events like user actions, sensor outputs, or messages from other systems. Each event triggers a specific action in response.
Explanation: This flow is highly dynamic and allows for real-time interaction with users or external systems, commonly used in UI programming and networked applications.
12. How does exception handling affect control flow?
Exception handling changes the flow of a program when an error occurs. Using constructs like try
, catch
, and finally
, developers can manage unexpected events and prevent crashes.
Explanation: Proper exception handling ensures the flow of the program continues or is gracefully terminated in the event of an error.
13. What are flow charts, and why are they used in programming?
Flow charts are graphical representations of a program’s flow, using symbols to illustrate steps, decision points, and processes. They help visualize complex algorithms and logic.
Explanation: Flow charts simplify understanding of how a program works, making it easier to design and debug processes.
14. What are finite state machines (FSMs), and how do they represent flow?
FSMs are computational models used to represent a system that can be in one of many predefined states. Transitions between states are determined by inputs or events, helping to model the flow of complex systems.
Explanation: FSMs provide a structured way to manage state transitions and flow in systems with well-defined states, such as games or network protocols.
15. Explain the concept of recursive flow.
Recursion is a flow control mechanism where a function calls itself to solve smaller instances of the same problem. The flow continues until a base condition is met, at which point the function returns and resolves each level of recursion.
Explanation: Recursive flow is useful for problems like tree traversal, but it can lead to excessive memory usage if not handled correctly.
Planning to Write a Resume?
Check our job winning resume samples
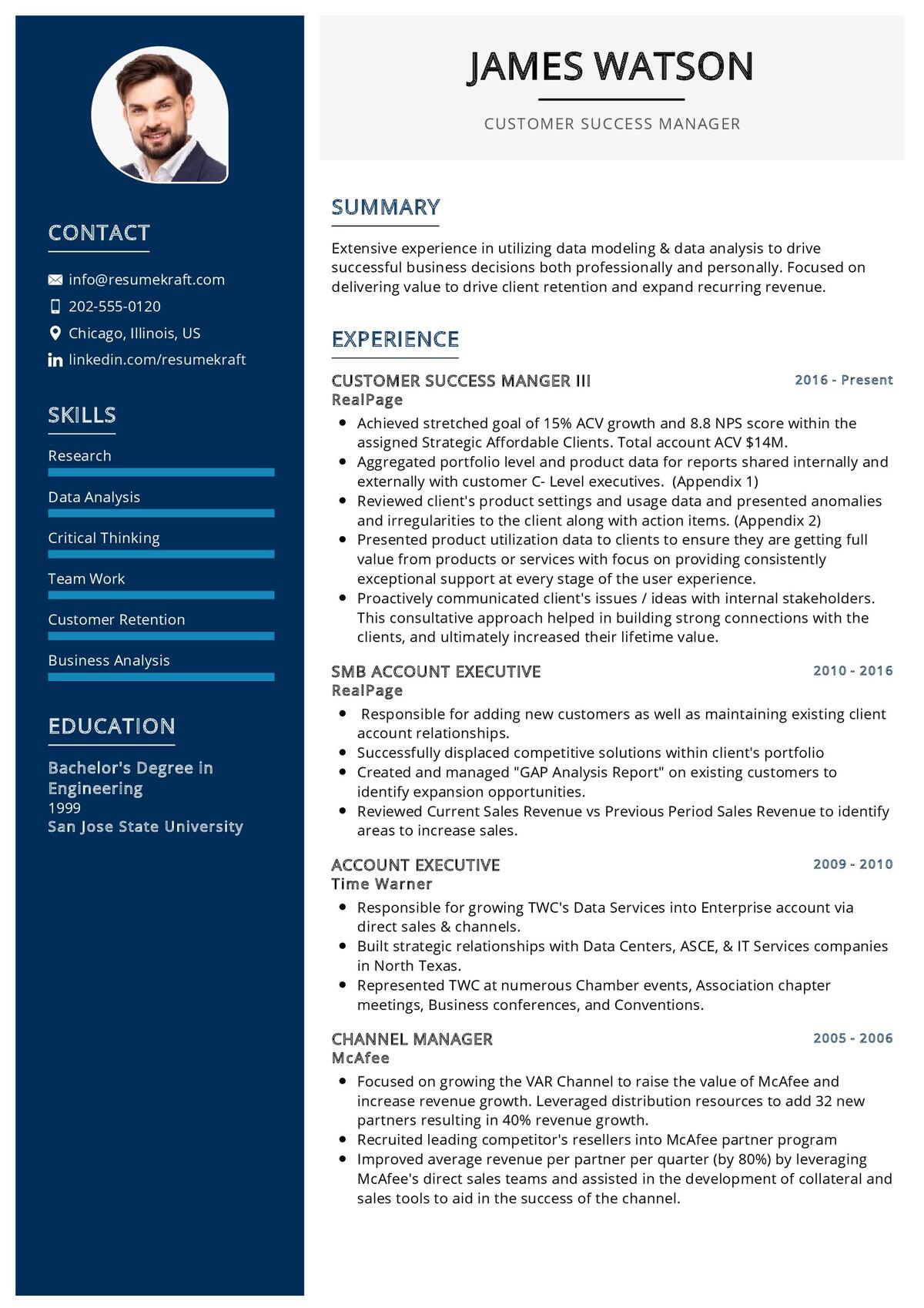
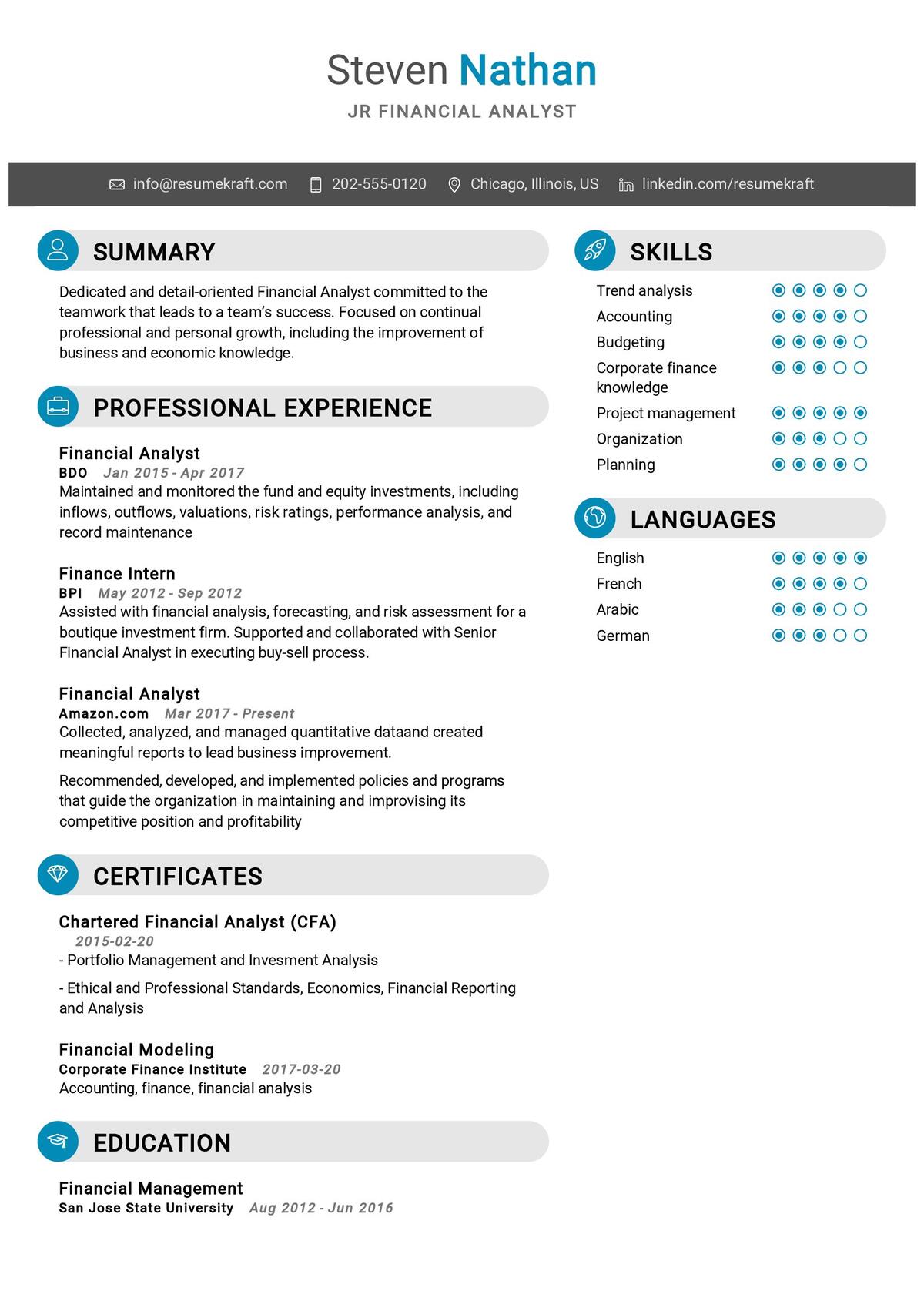
16. What is the difference between a stack and a queue in flow control?
A stack is a last-in-first-out (LIFO) data structure where elements are added and removed from the top. A queue is a first-in-first-out (FIFO) structure where elements are added to the back and removed from the front.
Explanation: Stacks and queues manage flow differently, with stacks being ideal for backtracking and queues for task scheduling.
17. What is flow control in networking?
Flow control in networking refers to the management of data transmission rates between devices to prevent a fast sender from overwhelming a slow receiver. Protocols like TCP implement flow control mechanisms.
Explanation: Flow control ensures that data is transmitted efficiently and reliably, avoiding packet loss and congestion in the network.
18. What is backpressure in flow control?
Backpressure is a technique used to manage the flow of data by slowing down or halting upstream producers when downstream consumers are overwhelmed. It’s commonly used in reactive programming.
Explanation: Backpressure prevents system overload by controlling the pace of data flow based on the processing capacity of each component.
19. How does the use of flow-based programming (FBP) affect system design?
FBP is a programming paradigm where applications are built by connecting independent processes via data flows. This approach simplifies the design and improves modularity and scalability.
Explanation: FBP encourages component reuse and allows easy scaling by managing the flow of data between processes.
20. How does the control flow differ in functional programming?
In functional programming, control flow is handled through recursion, higher-order functions, and function composition rather than using loops and conditional statements as in imperative programming.
Explanation: Functional programming treats control flow as a series of function evaluations, making it more predictable and mathematically sound.
21. What is reactive programming, and how does it affect flow?
Reactive programming is a declarative programming paradigm focused on the flow of data and propagation of changes. In reactive systems, the flow reacts to data streams and automatically updates based on new inputs.
Explanation: Reactive programming simplifies dealing with asynchronous data streams and events, allowing for more responsive systems.
22. Explain the flow of a REST API request.
In a REST API, the flow begins with a client sending an HTTP request to the server. The server processes the request, retrieves or manipulates data, and returns an HTTP response, which is then handled by the client.
Explanation: Understanding the flow of REST API requests is essential for building scalable web services that handle data retrieval and manipulation efficiently.
23. How does flow differ in an event loop in JavaScript?
The event loop in JavaScript manages the flow by continuously checking the call stack and callback queue. If the call stack is empty, it picks up tasks from the queue and processes them, allowing asynchronous tasks to be handled efficiently.
Explanation: The event loop ensures a smooth flow of asynchronous operations in JavaScript, making it non-blocking and event-driven.
24. What is parallel flow, and how does it differ from
concurrent flow?**
Parallel flow refers to executing multiple operations simultaneously on different processors or cores. Concurrent flow involves managing multiple tasks that make progress over time, not necessarily at the same instant.
Explanation: Parallel flow achieves real-time simultaneous execution, while concurrent flow focuses on efficient task switching to manage time.
25. What is pipeline flow, and where is it commonly used?
Pipeline flow refers to processing data in stages, where the output of one stage serves as the input for the next. This method is commonly used in data processing systems like ETL pipelines.
Explanation: Pipeline flow helps organize tasks into efficient stages, allowing for concurrent processing of different stages, which improves system throughput.
26. How do flow control mechanisms in Java affect multithreading?
Java offers several flow control mechanisms such as wait()
, notify()
, and notifyAll()
for managing the flow of data between multiple threads. These mechanisms help prevent race conditions and ensure synchronized access to shared resources.
Explanation: Proper flow control in multithreading prevents deadlock and ensures that threads operate in a coordinated manner, maintaining system stability.
27. What is flow testing in software development?
Flow testing is a type of testing focused on validating the control and data flow of an application. It ensures that the program’s flow paths execute correctly and that data is handled properly throughout the process.
Explanation: Flow testing ensures that applications behave as expected in different scenarios, preventing logical errors and data handling issues.
Conclusion
Flow control is a fundamental concept in programming and system design, ensuring that tasks are executed in the correct sequence and that data moves efficiently through a system. Mastering flow concepts is essential for solving complex problems, improving performance, and building scalable, maintainable systems. The 27 interview questions covered in this article provide a solid foundation for understanding how flow is managed across different programming paradigms, asynchronous operations, and system architectures. By preparing for these questions, you can demonstrate your knowledge of flow control, asynchronous programming, and system design, which are critical skills for any software development role.
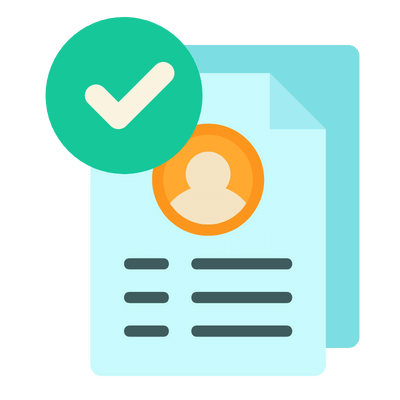
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
Recommended Reading: