As one of the leading companies in the defense, infrastructure, and technology sectors, KBR (Kellogg Brown & Root) offers a challenging and rewarding career path for software engineers. If you’re preparing for a software engineering interview with KBR, it’s essential to have a strong foundation in both technical knowledge and problem-solving skills. This article will guide you through the most common questions you may encounter during the interview process and help you prepare effectively. These questions focus on various aspects of software engineering, from coding to problem-solving and system design.
Top 34 KBR Software Engineer Interview Questions
1. What is the difference between object-oriented programming and procedural programming?
Object-oriented programming (OOP) is based on the concept of objects, which can hold data and methods, while procedural programming focuses on a step-by-step procedure to execute a task. OOP encourages the use of concepts like inheritance, encapsulation, and polymorphism, making code reusable and easier to maintain. In contrast, procedural programming relies more on a sequence of commands and is often less modular.
Explanation:
Object-oriented programming organizes code into reusable objects, improving code readability and maintenance, whereas procedural programming relies on sequential instructions.
2. Can you explain the concept of encapsulation in OOP?
Encapsulation is one of the core principles of object-oriented programming. It refers to the bundling of data (variables) and methods (functions) that operate on the data into a single unit, or class. By using encapsulation, you can control access to the data, restricting direct access to the inner workings of objects and promoting data security.
Explanation:
Encapsulation helps in protecting the data by providing controlled access through public methods and hiding the internal implementation.
3. How does a binary search algorithm work?
A binary search algorithm works by repeatedly dividing a sorted array in half, comparing the middle element to the target value. If the target value is equal to the middle element, the search is successful. If the target value is smaller, the search continues in the lower half, and if larger, in the upper half. This process repeats until the value is found or the search space is reduced to zero.
Explanation:
Binary search is an efficient algorithm with a time complexity of O(log n) due to its divide-and-conquer approach.
4. What are the main differences between SQL and NoSQL databases?
SQL databases are relational and structured, using tables to store data with fixed schemas. They follow ACID (Atomicity, Consistency, Isolation, Durability) properties. NoSQL databases are non-relational and more flexible, often schema-less, making them suitable for unstructured or semi-structured data. NoSQL databases prioritize scalability and availability over strict consistency.
Explanation:
SQL databases are ideal for structured data and complex queries, while NoSQL databases are designed for scalability and handling large volumes of unstructured data.
5. What is a microservices architecture?
Microservices architecture is a software development approach where an application is composed of small, independent services that communicate via APIs. Each microservice is responsible for a specific functionality and can be deployed, scaled, and updated independently. This architecture promotes flexibility, scalability, and easy maintenance of large applications.
Explanation:
Microservices allow for independent development and scaling, improving the efficiency and reliability of software systems.
6. How would you handle error logging in a distributed system?
In a distributed system, error logging needs to be centralized to ensure proper tracking across various services. This can be achieved by using logging frameworks or tools like ELK (Elasticsearch, Logstash, Kibana) or Graylog, which aggregate logs from multiple services. Proper error handling and notifications should be implemented to detect issues quickly.
Explanation:
Centralized logging ensures that errors in a distributed system are tracked and resolved efficiently, reducing downtime.
7. Can you explain the concept of inheritance in OOP?
Inheritance allows one class to acquire the properties and behaviors (methods) of another class. It enables code reuse and creates a relationship between parent (base) and child (derived) classes. The derived class inherits all the accessible attributes and methods from the parent class but can also have its own unique properties.
Explanation:
Inheritance facilitates code reuse by allowing derived classes to inherit common functionality from base classes.
8. What is the difference between synchronous and asynchronous programming?
In synchronous programming, tasks are executed sequentially, meaning each task must complete before the next begins. Asynchronous programming allows multiple tasks to run concurrently, where tasks can start without waiting for others to complete. This improves performance, especially in I/O-bound operations.
Explanation:
Asynchronous programming improves efficiency by allowing tasks to run concurrently, preventing blocking operations.
Build your resume in just 5 minutes with AI.
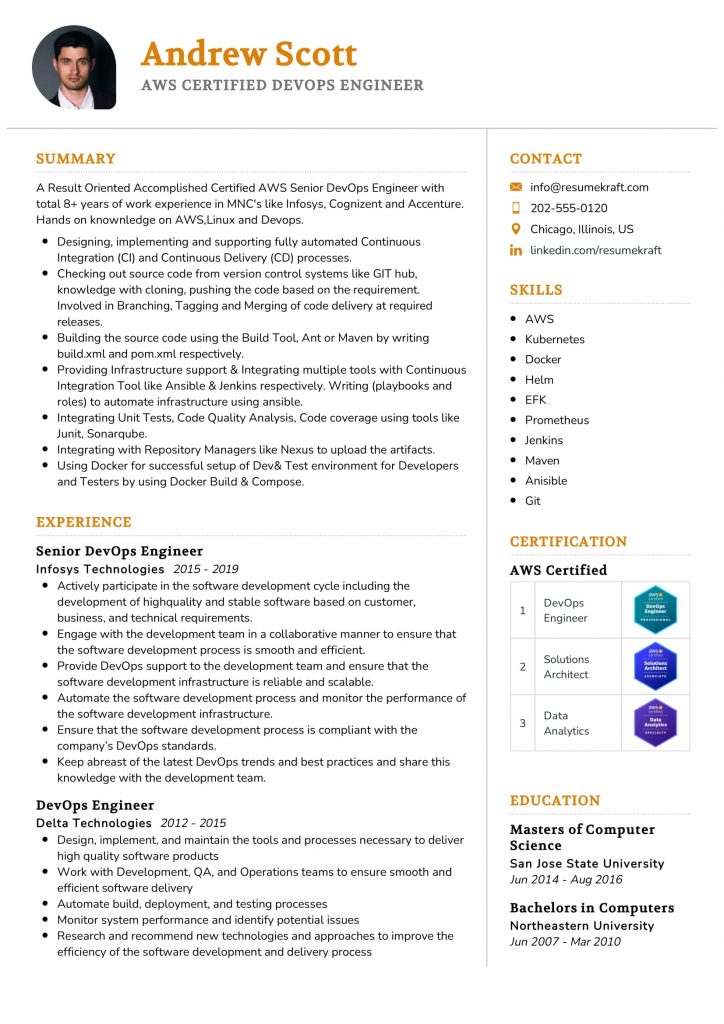
9. How does garbage collection work in Java?
Garbage collection in Java automatically frees up memory by identifying and disposing of objects that are no longer in use. The Java Virtual Machine (JVM) runs the garbage collector, which uses algorithms like mark-and-sweep or generational garbage collection to reclaim memory. This prevents memory leaks and ensures efficient memory management.
Explanation:
Garbage collection helps in managing memory automatically, reducing the chances of memory leaks and improving application performance.
10. What is the difference between REST and SOAP APIs?
REST (Representational State Transfer) is a lightweight, scalable API protocol that uses HTTP methods and JSON/XML for communication. SOAP (Simple Object Access Protocol) is more rigid and uses XML for data exchange. REST is stateless and more flexible, while SOAP provides built-in error handling and security features like WS-Security.
Explanation:
REST is preferred for web services due to its simplicity and scalability, while SOAP offers more security and error handling for enterprise-level applications.
11. What are design patterns, and why are they important?
Design patterns are reusable solutions to common software design problems. They provide best practices to solve recurring challenges in software development. Using design patterns improves code maintainability, readability, and reusability by promoting standard solutions.
Explanation:
Design patterns streamline the development process by offering proven solutions for common problems, making code easier to manage and scale.
12. Can you explain the concept of polymorphism in OOP?
Polymorphism allows objects of different classes to be treated as instances of the same class through a common interface. It enables a single method or function to operate in different ways, depending on the object it is acting upon. Polymorphism is achieved through method overriding and method overloading.
Explanation:
Polymorphism increases flexibility in code by allowing the same interface to handle different types of objects and behaviors.
13. How would you optimize a slow SQL query?
To optimize a slow SQL query, you can start by analyzing the query plan using tools like EXPLAIN or ANALYZE. Indexes should be used on frequently queried columns to speed up lookups. Avoid using SELECT *, and prefer limiting data retrieval with WHERE clauses. Additionally, normalization and optimizing joins can improve performance.
Explanation:
Query optimization techniques like indexing, query restructuring, and limiting data retrieval can significantly reduce execution time.
14. What is the purpose of version control systems like Git?
Version control systems like Git help developers track changes to code over time, enabling collaboration and preventing conflicts. Git allows you to revert to previous versions, work on branches, and merge changes from multiple contributors seamlessly. It ensures that code history is preserved and manageable.
Explanation:
Version control systems facilitate teamwork by allowing multiple developers to work on the same codebase while maintaining a history of changes.
15. How would you design a scalable web application?
To design a scalable web application, you can start by using load balancing to distribute traffic across multiple servers. Database optimization, such as sharding or replication, can also improve scalability. Implementing microservices architecture, caching frequently accessed data, and utilizing cloud infrastructure are other effective strategies.
Explanation:
Scalable web applications distribute workloads efficiently and can handle increasing user demand by using techniques like load balancing and caching.
16. What is the difference between a thread and a process?
A thread is the smallest unit of execution within a process, while a process is a self-contained program that runs in its own memory space. Multiple threads can exist within a process and share the same memory, whereas processes are isolated and do not share memory. Threads are lighter and faster than processes.
Explanation:
Threads allow for concurrent execution within a process, sharing memory, while processes are independent and have their own memory space.
17. Can you explain dependency injection?
Dependency injection is a design pattern in which an object’s dependencies are provided to it from the outside rather than being created within the object. This promotes loose coupling, making the code more flexible and testable. Common frameworks like Spring and Angular use dependency injection to manage object lifecycles.
Explanation:
Dependency injection improves code maintainability by separating object creation from its usage, enhancing flexibility and testability.
18. What is the purpose of the SOLID principles?
The SOLID principles are a set of five design guidelines that aim to make software design more understandable, flexible, and maintainable. These principles include Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. Following SOLID principles leads to more robust and scalable software.
Explanation:
The SOLID principles promote better software design, reducing complexity and increasing the flexibility and robustness of the code.
19. How do you manage state in a React application?
In a React application, state can be managed using
the built-in useState
hook for local component state or more advanced tools like Redux for global state management. Context API is another option to manage state across multiple components without prop drilling. Proper state management ensures predictable UI behavior.
Explanation:
State management in React ensures that the application behaves consistently as user interactions and data changes occur.
20. What are the benefits of using Docker for software development?
Docker enables developers to create, deploy, and run applications in isolated containers. This ensures that applications behave the same in different environments by packaging all dependencies into a single container. Docker enhances collaboration, scalability, and security while reducing deployment errors.
Explanation:
Docker containers promote consistency across environments, improving development efficiency and reducing issues related to dependency management.
21. Can you explain how caching works?
Caching is a technique used to store frequently accessed data in a temporary storage area, so it can be retrieved quickly without recalculating or fetching it from the database. Caching can be implemented at various levels, including browser cache, server-side cache, and CDN cache. This reduces load times and improves application performance.
Explanation:
Caching speeds up data retrieval by storing frequently used data temporarily, reducing the need for repetitive computations or database queries.
22. How does an API gateway work in a microservices architecture?
An API gateway acts as a single entry point for clients to access multiple services in a microservices architecture. It routes requests to the appropriate service, handles authentication, and can perform other tasks like rate limiting and caching. The API gateway simplifies the client interface and centralizes service management.
Explanation:
An API gateway streamlines communication between clients and microservices, providing a centralized access point and improving security and scalability.
23. What are the key differences between HTTP and HTTPS?
HTTP (Hypertext Transfer Protocol) is the standard protocol for transmitting data over the web, while HTTPS (HTTP Secure) adds an extra layer of security by encrypting the communication using SSL/TLS. HTTPS ensures that data transferred between the client and server is secure and protected from eavesdropping or tampering.
Explanation:
HTTPS provides encrypted communication, ensuring that sensitive data transmitted over the web is secure and protected from malicious attacks.
24. Can you explain the concept of a load balancer?
A load balancer distributes incoming network traffic across multiple servers to ensure no single server is overwhelmed. This improves the availability, reliability, and scalability of applications by balancing the workload. Load balancers can work at different layers of the network stack, such as Layer 4 (Transport) or Layer 7 (Application).
Explanation:
Load balancers enhance the performance and availability of applications by distributing traffic evenly across multiple servers.
25. What is the difference between continuous integration and continuous deployment?
Continuous integration (CI) involves regularly merging code changes into a shared repository and automatically testing the integration. Continuous deployment (CD) extends CI by automating the deployment of code to production after passing all tests. CI ensures code integration, while CD ensures that the latest changes are always live.
Explanation:
Continuous integration and continuous deployment streamline the development process by automating code testing and deployment, reducing the risk of errors.
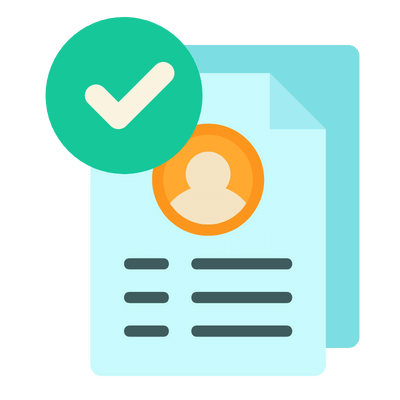
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
26. How would you handle security in a web application?
To handle security in a web application, you should implement measures like HTTPS, input validation, and output encoding to prevent attacks like SQL injection and XSS. Use proper authentication and authorization protocols such as OAuth 2.0 and secure session management. Implement regular security audits and encryption for sensitive data.
Explanation:
Ensuring web application security requires proactive measures like input validation, encryption, and regular security assessments.
27. What are the differences between GET and POST HTTP methods?
The GET method retrieves data from a server and appends parameters to the URL, making it less secure for sensitive data. The POST method sends data in the request body, making it more secure for sending sensitive or large amounts of data. GET requests can be cached, while POST requests cannot.
Explanation:
GET is used for retrieving data and is visible in the URL, while POST is used for sending data securely in the request body.
28. What is the purpose of middleware in a web application?
Middleware acts as a layer between the request and response in a web application. It handles tasks like authentication, logging, request parsing, and error handling. Middleware allows for modular and reusable code by performing common tasks across multiple routes or endpoints.
Explanation:
Middleware simplifies common web application tasks by creating reusable layers that can handle requests before reaching the main application logic.
29. Can you explain the difference between unit testing and integration testing?
Unit testing focuses on testing individual components or functions of a program in isolation to ensure they work as expected. Integration testing, on the other hand, tests the interaction between different components or systems to ensure they function together correctly. Both are essential for a robust testing strategy.
Explanation:
Unit testing verifies individual components in isolation, while integration testing ensures that different components work well together.
30. How does OAuth 2.0 work for authorization?
OAuth 2.0 is an authorization protocol that allows third-party services to access user resources without sharing credentials. It works by issuing tokens to authorized clients, which can then be used to access protected resources. OAuth 2.0 supports various flows like Authorization Code, Implicit, and Client Credentials.
Explanation:
OAuth 2.0 enables secure authorization by issuing tokens, allowing third-party services to access resources without exposing user credentials.
31. What is the purpose of rate limiting in web applications?
Rate limiting is a technique used to control the number of requests a client can make to a server within a specific time frame. It helps prevent abuse, such as DDoS attacks or excessive API calls, ensuring the server remains available to legitimate users. Rate limiting can be implemented using API gateways or middleware.
Explanation:
Rate limiting protects web applications from being overwhelmed by excessive requests, ensuring availability and preventing misuse.
32. Can you explain the concept of a monolithic architecture?
Monolithic architecture is a traditional software design where all components of an application are tightly coupled and run as a single unit. While easier to develop and deploy initially, monolithic architectures can become difficult to scale and maintain as applications grow in complexity.
Explanation:
Monolithic architectures group all components into a single unit, making them less scalable and harder to maintain as the system grows.
33. What is event-driven programming?
Event-driven programming is a paradigm where the flow of the program is determined by events, such as user interactions, sensor outputs, or messages from other programs. It is commonly used in graphical user interfaces and web applications where the system reacts to events like clicks or keyboard input.
Explanation:
Event-driven programming responds to user actions and other events, enabling interactive and dynamic applications.
34. How do you ensure code quality in a software project?
To ensure code quality, you should adopt practices such as writing clean, maintainable code, conducting code reviews, and following coding standards. Automated testing, continuous integration, and static code analysis tools also help identify and fix issues early in the development process.
Explanation:
Maintaining high code quality involves best practices like code reviews, testing, and using tools to detect issues early in development.
Conclusion
Preparing for a KBR software engineer interview requires a solid grasp of both technical and problem-solving skills. By reviewing these top 34 questions and understanding the concepts behind them, you’ll be better equipped to handle the interview with confidence. Whether you’re focusing on coding, system design, or software architecture, mastering these topics will give you a competitive edge.
If you’re preparing for interviews or updating your resume, check out these resume examples, or explore our resume builder to craft a professional resume effortlessly. You can also download free resume templates to get started today.
Recommended Reading: