Pytest is a popular testing framework in Python, known for its simplicity, flexibility, and support for test-driven development (TDD). Whether you’re testing a large software system or a simple script, Pytest provides the tools to write, manage, and automate your tests effectively. For professionals looking to secure a role involving Python development, being proficient in Pytest is essential. In this article, we present the top 37 Pytest interview questions that will help you prepare for your upcoming interview, covering a range of fundamental and advanced topics.
Top 37 Pytest Interview Questions
1. What is Pytest, and why is it used?
Pytest is a Python testing framework used to write and execute test cases efficiently. It supports simple unit tests and complex functional testing. One of its strengths is that it can automatically discover tests based on file names and functions.
Explanation
Pytest is preferred because it is easy to use and supports features like fixtures, parameterized tests, and plugins, making it ideal for both small and large projects.
2. How do you install Pytest?
You can install Pytest using the following command:
pip install pytest
This will install Pytest and its dependencies, allowing you to run tests by using the pytest
command.
Explanation
Installing Pytest is straightforward using Python’s package manager, pip
. It is compatible with various Python versions, including 3.x and newer.
3. How do you write a basic test case using Pytest?
To write a basic test case, create a Python function starting with test_
. Inside this function, use assertions to validate the expected outcome.
def test_example():
assert 1 + 1 == 2
Running pytest
in the terminal will automatically discover and run this test.
Explanation
Pytest automatically identifies test functions by looking for those prefixed with test_
. The framework runs these and checks for assertion failures.
4. How does Pytest differ from the unittest
framework?
Pytest is simpler and more concise compared to unittest
. It does not require boilerplate code like setUp
and tearDown
, and it supports advanced features like fixtures, parameterization, and plugins, making it more flexible.
Explanation
Pytest is widely preferred for its minimalistic approach and advanced testing features. unittest
, though robust, is more verbose and less flexible in certain cases.
5. What are fixtures in Pytest?
Fixtures in Pytest are used to set up preconditions before a test runs, like initializing database connections or preparing test data. Fixtures can be shared across multiple tests using the @pytest.fixture
decorator.
Explanation
Fixtures are essential for managing complex test setups. They provide reusable setups that reduce code duplication and improve test organization.
6. How do you use fixtures in Pytest?
To use a fixture, define it with the @pytest.fixture
decorator and then pass the fixture name as an argument in your test function. Pytest will automatically inject the fixture’s return value into the test.
Explanation
Fixtures are automatically managed by Pytest, making it easy to share setup code across tests. This encourages clean, maintainable test code.
7. Can Pytest run unittest
-style tests?
Yes, Pytest is compatible with unittest
. It can run test cases written using the unittest
framework. This makes Pytest a versatile tool that can be adopted in legacy codebases.
Explanation
Pytest’s ability to run unittest
-style tests allows for gradual migration from older testing frameworks without breaking existing test suites.
Build your resume in just 5 minutes with AI.
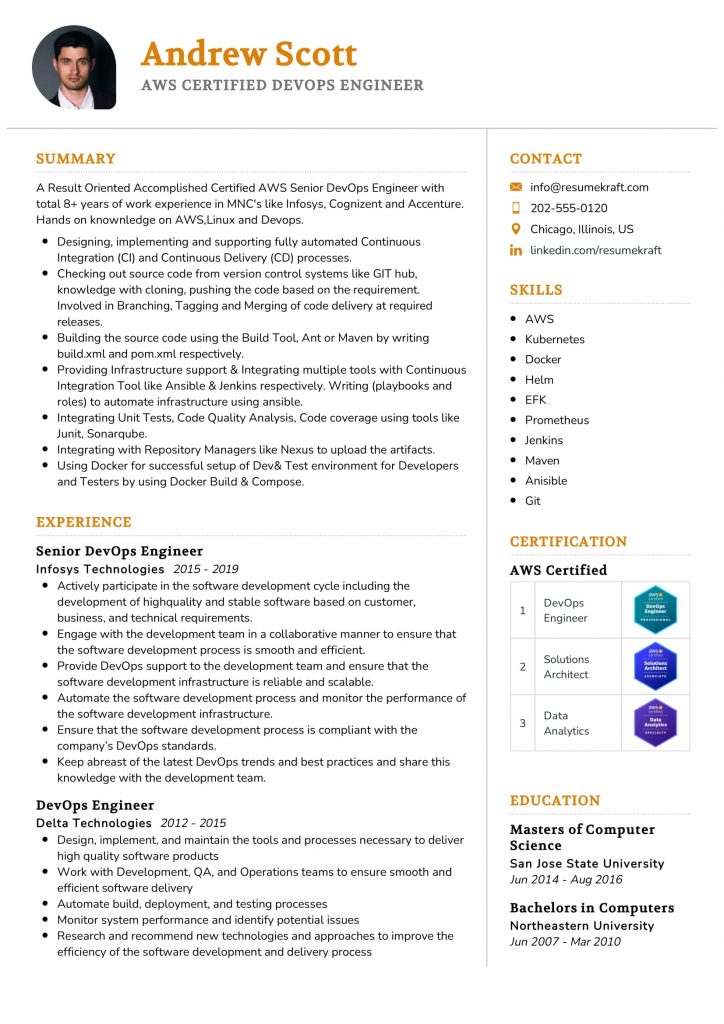
8. How can you parametrize tests in Pytest?
Pytest allows parameterization of tests using the @pytest.mark.parametrize
decorator. This allows you to run a test with different sets of input data.
@pytest.mark.parametrize("input,expected", [(1, 2), (2, 3), (3, 4)])
def test_increment(input, expected):
assert input + 1 == expected
Explanation
Parameterized tests help reduce redundancy by running the same test logic with multiple inputs, increasing test coverage with minimal code.
9. How can you skip a test in Pytest?
You can skip a test using the @pytest.mark.skip
decorator or dynamically skipping within a test by using the pytest.skip()
function.
Explanation
Skipping tests is useful when certain conditions make a test irrelevant or when features are not yet implemented.
10. What is the purpose of @pytest.mark.xfail
?
The @pytest.mark.xfail
decorator is used to mark tests that are expected to fail due to known issues. Pytest will report these tests as “expected failures” without marking the overall test run as failed.
Explanation
Using xfail
helps in tracking known issues without affecting the overall test suite’s success rate.
11. How do you group tests in Pytest?
Tests can be grouped using markers. You can define custom markers using the @pytest.mark.<name>
decorator, and then run a specific group of tests with the -m
option.
pytest -m "group1"
Explanation
Grouping tests via markers allows for selective execution of tests, useful in large projects where you want to run only specific categories of tests.
12. How can you run only failed tests in Pytest?
Pytest provides the --lf
(last failed) option, which reruns only the tests that failed in the previous run.
pytest --lf
Explanation
This feature is handy for debugging purposes, allowing developers to focus on fixing failed tests without running the entire test suite.
13. What is Pytest’s plugin architecture?
Pytest supports a rich plugin architecture, allowing users to extend its functionality. You can install third-party plugins or create custom plugins for specific use cases.
Explanation
The plugin architecture makes Pytest extremely customizable, giving it flexibility for various testing needs across different projects.
14. What is conftest.py
in Pytest?
conftest.py
is a special configuration file used in Pytest to define fixtures or hooks that are shared across multiple test files in a directory.
Explanation
Using conftest.py
, you can avoid redundant fixture imports and centralize configurations, ensuring better organization and maintenance.
15. How do you capture output in Pytest?
You can capture standard output using the capsys
or caplog
fixtures provided by Pytest. These allow you to assert what was printed or logged during a test.
Explanation
Capturing output is essential when testing code that prints or logs information. Pytest provides built-in fixtures to handle this easily.
16. How do you run Pytest with coverage?
To run Pytest with coverage, you can install the pytest-cov
plugin and run Pytest with the --cov
option:
pytest --cov=<module_name>
Explanation
Code coverage helps identify untested code paths, ensuring that your tests provide sufficient coverage of your application.
17. How do you handle exceptions in Pytest?
Pytest provides the pytest.raises()
context manager to test code that is expected to raise exceptions.
with pytest.raises(ValueError):
raise ValueError("Invalid input")
Explanation
Testing exceptions ensures that your code handles error conditions properly. Pytest makes it easy to check for specific exceptions.
18. What are Pytest hooks?
Hooks in Pytest are special functions that can alter the behavior of the test runner at different points during the test execution lifecycle. For example, pytest_runtest_setup
is a hook that runs before each test.
Explanation
Hooks allow you to extend or modify the default behavior of Pytest, providing a powerful way to integrate custom actions during test execution.
19. How do you run Pytest tests in parallel?
You can run Pytest tests in parallel by using the pytest-xdist
plugin. Install it via pip install pytest-xdist
and run tests with the -n
option:
pytest -n 4
Explanation
Running tests in parallel reduces overall test execution time, especially for large test suites, improving efficiency.
20. How do you mark a test as slow in Pytest?
You can create a custom marker like slow
and apply it to slow tests. Then, you can run or skip these tests based on your preferences.
@pytest.mark.slow
def test_slow_function():
pass
Explanation
Marking slow tests helps in selectively running them when needed, without affecting the speed of regular test runs.
21. How do you assert that a warning is raised in Pytest?
Pytest provides the recwarn
fixture to capture and assert warnings raised during a test.
def test_warning(recwarn):
with pytest.warns(UserWarning):
warnings.warn("This is a warning", UserWarning)
Explanation
Warning assertions ensure that your code behaves as expected when non-critical issues are raised.
22. What is the purpose of pytest.ini
?
pytest.ini
is a configuration file that stores Pytest settings, such as custom markers or command-line options. This
allows you to manage test settings centrally.
Explanation
Using pytest.ini
simplifies the process of configuring tests across your entire project, avoiding the need for repetitive command-line arguments.
23. Can you use Pytest with Django?
Yes, Pytest can be integrated with Django using the pytest-django
plugin. This allows for seamless testing of Django models, views, and templates.
Explanation
Pytest’s compatibility with Django makes it a powerful tool for testing full-stack web applications, providing better testing flexibility than Django’s built-in testing framework.
24. What is the -k
option in Pytest?
The -k
option allows you to run tests that match a specific expression or substring in the test names.
pytest -k "test_example"
Explanation
The -k
option is useful when you want to run a subset of tests that match certain keywords, speeding up test selection.
25. How can you stop the test run after the first failure?
Use the -x
option to stop the test execution after the first failure.
pytest -x
Explanation
This is helpful when debugging, as it allows you to address issues one at a time without waiting for the full test suite to run.
26. What are Pytest fixtures’ scopes?
Fixture scopes define how often a fixture is set up and torn down. Common scopes include function
, class
, module
, and session
. A function
scope fixture runs before each test function, while a session
scope fixture runs once for the entire test session.
Explanation
Choosing the correct fixture scope can optimize test execution by reducing unnecessary setup and teardown operations.
27. How do you debug failing tests in Pytest?
You can use the --pdb
option to drop into Python’s debugger when a test fails. This allows you to inspect the state of variables and understand the cause of failure.
Explanation
Using Pytest’s built-in debugging options helps you quickly identify and resolve issues during testing.
28. How do you test command-line scripts with Pytest?
You can test command-line scripts by using the subprocess
module or pytester
fixture provided by Pytest. These allow you to simulate command-line executions and assert outputs.
Explanation
Command-line scripts are an essential part of many applications, and Pytest makes it easy to test them as part of your test suite.
29. How do you rerun failed tests in Pytest?
The pytest-rerunfailures
plugin allows you to automatically rerun failed tests a specified number of times before marking them as failed.
pytest --reruns 3
Explanation
Rerunning failed tests helps eliminate intermittent issues, such as network glitches or timing issues, which could cause spurious test failures.
30. What is the --maxfail
option in Pytest?
The --maxfail
option stops test execution after a certain number of failures. This helps save time by preventing the entire test suite from running when multiple failures occur.
pytest --maxfail=2
Explanation
This option helps developers focus on critical issues rather than waiting for the entire test suite to fail when debugging multiple errors.
31. How can you test logging in Pytest?
You can test logging using the caplog
fixture. This fixture captures logs during test execution, allowing you to make assertions about log content.
def test_logging(caplog):
logger = logging.getLogger()
logger.error("An error occurred")
assert "An error occurred" in caplog.text
Explanation
Testing logs ensures that your application provides the necessary information for debugging and monitoring in production environments.
32. How do you test APIs using Pytest?
You can test APIs in Pytest by using Python’s requests
module to make HTTP calls, then asserting the response data and status codes.
def test_api():
response = requests.get('https://api.example.com/data')
assert response.status_code == 200
Explanation
API testing is critical in modern applications. Pytest, combined with requests
, offers an efficient way to test both external and internal APIs.
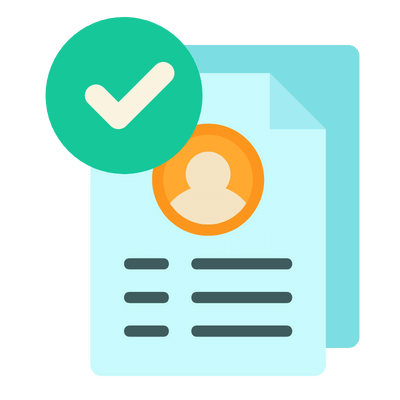
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
33. How do you handle database tests in Pytest?
For database tests, Pytest can be combined with fixtures to set up and tear down a test database. For example, in Django, you can use the pytest-django
plugin to manage test databases.
Explanation
Testing databases requires careful management of data states. Pytest’s fixtures simplify setting up isolated test environments.
34. How do you create a custom marker in Pytest?
To create a custom marker, define it in your pytest.ini
file and then use it in your tests with the @pytest.mark.<marker_name>
decorator.
[pytest]
markers =
slow: marks tests as slow
Explanation
Custom markers allow you to categorize tests beyond the default options provided by Pytest, improving test suite management.
35. How do you generate test reports in Pytest?
You can generate test reports in HTML format using the pytest-html
plugin. Install it using pip install pytest-html
and run Pytest with the --html
option:
pytest --html=report.html
Explanation
Test reports provide a detailed summary of test results, making it easier to review failures and successes in large test suites.
36. What is Pytest’s monkeypatch
fixture?
The monkeypatch
fixture allows you to modify or mock attributes, methods, or environment variables in tests. This is useful when testing components that depend on external factors.
def test_monkeypatch(monkeypatch):
monkeypatch.setattr('os.getenv', lambda key: 'fake_value')
assert os.getenv('SOME_KEY') == 'fake_value'
Explanation
Monkeypatching helps isolate the code under test from dependencies, making it easier to test different behaviors and edge cases.
37. How do you test asynchronous code with Pytest?
You can test asynchronous code by using the pytest-asyncio
plugin. This allows you to define async
test functions and await asynchronous code.
@pytest.mark.asyncio
async def test_async_function():
result = await some_async_function()
assert result == expected_value
Explanation
With the rise of asynchronous programming in Python, Pytest’s ability to handle async tests ensures you can validate modern, non-blocking code patterns.
Conclusion
Pytest is a versatile and powerful testing framework that supports a wide range of testing needs in Python. Whether you’re working on simple scripts or large-scale applications, understanding the basics of Pytest will give you an edge in interviews and daily development tasks. We’ve covered the top 37 Pytest interview questions to help you get started or solidify your knowledge. For further career development, consider utilizing tools like our resume builder to present your skills and achievements effectively. Explore free resume templates and resume examples to create a resume that stands out in today’s competitive job market.
Recommended Reading: