Salesforce Apex is an essential programming language for developers working within the Salesforce ecosystem. It enables developers to execute custom logic and interact with Salesforce data, creating powerful, robust applications. As Apex is strongly typed and object-oriented, it integrates seamlessly with Salesforce’s existing features, making it crucial for any developer who wishes to thrive in the Salesforce domain. This article provides a detailed list of the top Salesforce Apex interview questions to help you prepare for your next interview and excel in your Salesforce developer career.
Top 37 Salesforce Apex Interview Questions
1. What is Apex in Salesforce?
Apex is a proprietary programming language by Salesforce, specifically designed to interact with Salesforce objects. It allows developers to add custom business logic and create advanced features on top of the standard Salesforce functionalities.
Explanation: Apex runs in a multitenant environment, meaning the platform is shared by different organizations. It is compiled, stored, and executed in Salesforce’s cloud environment.
2. What are Apex Triggers?
Apex Triggers are pieces of code that execute before or after certain events, such as insert, update, delete, or undelete operations on Salesforce records. Triggers help in automating specific processes when a record is modified.
Explanation: Triggers are very powerful in enforcing data integrity and business processes across records, ensuring consistent execution of logic.
3. What is the difference between a Before Trigger and an After Trigger?
A Before Trigger allows you to make changes to a record before it is saved to the database. An After Trigger, on the other hand, allows you to access the record after it has been saved, but any changes to the record will not be saved.
Explanation: Before Triggers are used for data validation and manipulation, while After Triggers are typically used for sending notifications or updating related records.
4. What are Governor Limits in Apex?
Governor Limits are rules enforced by the Salesforce platform to ensure efficient use of shared resources. These limits restrict the amount of CPU time, memory, database queries, and records that a single execution context can use.
Explanation: Governor Limits help prevent excessive resource consumption, which could degrade performance for other users in the shared environment.
5. What is a SOQL query?
SOQL (Salesforce Object Query Language) is a query language used to retrieve records from Salesforce objects. It is similar to SQL but customized for Salesforce data structures.
Explanation: SOQL allows developers to query Salesforce databases for specific records, ensuring efficient data retrieval.
6. How do DML operations work in Apex?
DML (Data Manipulation Language) operations allow developers to insert, update, delete, or upsert records in the Salesforce database. These operations modify the database directly from the code.
Explanation: DML operations are essential for manipulating Salesforce data and implementing custom business logic in Apex.
7. What is a Bulk Trigger in Salesforce?
A Bulk Trigger is designed to handle large volumes of records efficiently. Apex automatically processes records in batches, which is why triggers need to be bulkified to handle multiple records simultaneously.
Explanation: Bulk triggers are necessary to ensure that the code complies with Salesforce’s governor limits and handles large datasets properly.
8. How can you prevent SOQL injection in Apex?
To prevent SOQL injection, it’s essential to use bind variables instead of concatenating strings directly in the SOQL query. Bind variables safely pass user input into a query, reducing the risk of injection attacks.
Explanation: Preventing SOQL injection is crucial for securing Salesforce applications from potential vulnerabilities.
9. What are Apex Collections?
Apex Collections are data structures like Lists, Sets, and Maps that allow developers to store multiple data types together. Collections are used extensively in Salesforce to handle complex data structures.
Explanation: Apex Collections simplify data handling, making it easier to manipulate and organize large datasets efficiently.
Build your resume in just 5 minutes with AI.
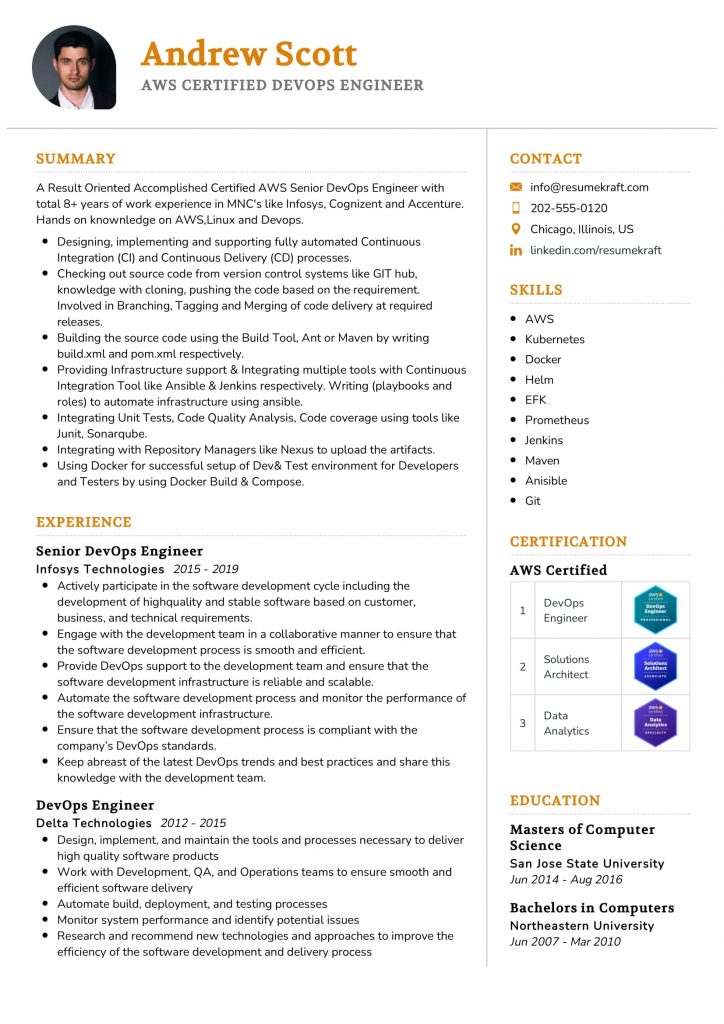
10. What are Apex Classes and Objects?
Apex Classes are user-defined blueprints or templates for creating objects. An object in Apex is an instance of a class that contains attributes and behaviors.
Explanation: Apex Classes and Objects form the foundation of object-oriented programming in Salesforce, enabling code reusability and organization.
11. How do you handle exceptions in Apex?
Apex uses try-catch blocks to handle exceptions. When an error occurs, the code inside the catch block is executed, ensuring that the application doesn’t crash.
Explanation: Exception handling is vital for writing robust and error-free applications in Salesforce.
12. What is the difference between a Map and a Set in Apex?
A Map stores data in key-value pairs, while a Set is an unordered collection of unique elements. Both are types of collections used for managing complex data structures.
Explanation: Maps are useful when you need to associate values with unique keys, whereas Sets are best when uniqueness is a priority.
13. What is the purpose of Test Classes in Apex?
Test Classes ensure that the code you write works as expected and adheres to Salesforce best practices. Every deployment to Salesforce requires a minimum of 75% code coverage from Test Classes.
Explanation: Test Classes are essential for validating code functionality and achieving successful deployments.
14. What are Custom Controllers in Salesforce?
Custom Controllers are Apex classes that allow developers to define custom logic and behavior for Visualforce pages. They give full control over the interaction between the page and Salesforce data.
Explanation: Custom Controllers provide flexibility in building custom Visualforce pages with complex business logic.
15. How do you optimize Apex code for performance?
Optimizing Apex code involves minimizing the number of SOQL queries, bulkifying triggers, and reducing the use of loops and DML statements within loops.
Explanation: Efficient Apex code ensures that applications run smoothly and within Salesforce’s governor limits.
16. What is Asynchronous Apex?
Asynchronous Apex allows developers to run processes in the background, freeing up resources and improving performance for long-running operations. This includes Batch Apex, Future methods, and Queueable Apex.
Explanation: Asynchronous Apex is crucial for handling time-intensive tasks that don’t need immediate execution.
17. What is a Future method in Salesforce?
A Future method is an asynchronous Apex method that runs in the background. It is often used for callouts to external services or for long-running operations.
Explanation: Future methods improve the responsiveness of applications by offloading resource-heavy tasks.
18. What is Batch Apex?
Batch Apex is used to process large volumes of data in chunks. It allows developers to break up operations into manageable batches, ensuring that they stay within governor limits.
Explanation: Batch Apex is necessary for processing thousands or millions of records efficiently without hitting governor limits.
19. What is Queueable Apex?
Queueable Apex is similar to Future methods but provides more control over the execution process. It allows chaining jobs and provides better monitoring of asynchronous operations.
Explanation: Queueable Apex is ideal for tasks that need more complex background processing than Future methods.
20. How do you use the @isTest annotation in Apex?
The @isTest annotation is used to define a test class or method. This ensures that the code is executed only during testing and does not affect production data.
Explanation: The @isTest annotation helps developers write test methods that validate the functionality of the application.
21. What is an Apex Managed Sharing Rule?
Apex Managed Sharing Rule allows developers to share records programmatically by creating a custom sharing rule. This is useful when standard sharing rules are insufficient.
Explanation: Apex Managed Sharing provides greater flexibility and control over who can access Salesforce records.
22. How do you prevent recursion in triggers?
Recursion in triggers can be prevented by using static variables. By checking if a static variable is true or false, you can ensure that the trigger doesn’t execute multiple times for the same record.
Explanation: Preventing recursion is crucial for avoiding infinite loops that can lead to governor limit violations.
23. What is the @future annotation?
The @future annotation is used to mark a method as asynchronous. This method runs in the background and is often used for operations like callouts to external services.
Explanation: The @future annotation enables developers to execute time-consuming tasks without affecting the user experience.
24. How can you debug Apex code?
Apex code can be debugged using debug logs and the System.debug() statement. The Salesforce Debug Logs capture every event during the execution of the code, helping developers identify issues.
Explanation: Effective debugging is essential for resolving issues and improving the functionality of Salesforce applications.
25. What is the use of the Database class in Apex?
The Database class in Apex provides additional DML operations, such as database.insert, that allow for partial success when inserting records. It also offers better control over transactions.
Explanation: The Database class is useful for handling DML operations more flexibly and controlling transaction behavior.
26. What is Governor Limit for SOQL queries?
Salesforce limits the number of SOQL queries you can execute in a single transaction to 100. This is to ensure that the shared Salesforce infrastructure remains stable and performant.
Explanation: Exceeding the governor limit can cause your application to throw an exception, so queries must be optimized.
27. What is the use of the Schema class?
The Schema class in Apex provides methods to get metadata about objects, fields, and relationships in Salesforce. This is useful for dynamic applications that need to interact with various Salesforce objects.
Explanation: The Schema class allows developers to build flexible applications that can interact with various Salesforce objects dynamically.
28. What is the difference between a Rollback and Commit?
A Rollback undoes all changes made in a transaction, whereas a Commit permanently saves the changes to the database. Rollbacks are used to revert transactions in case of errors.
Explanation: Proper use of rollback and commit ensures that database changes are only made when the operation is successful.
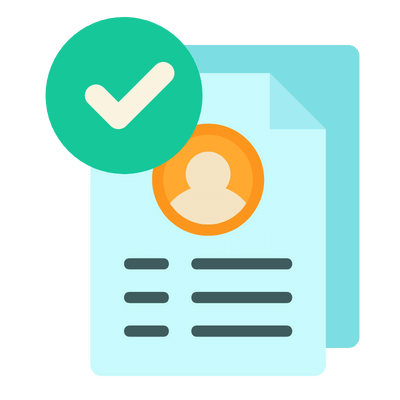
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
29. What are Custom Settings?
Custom Settings are similar to custom objects but are designed to store configuration data. They allow developers to define and manage application settings without hardcoding values.
Explanation: Custom Settings make applications more flexible and easier to maintain by storing configuration data outside of the code.
30. What is Dynamic Apex?
Dynamic Apex allows developers to write flexible code that can adapt to changes in the data model. This includes querying objects dynamically and using describe methods to interact with metadata.
Explanation: Dynamic Apex is powerful for applications that need to be adaptable and interact with various Salesforce objects.
31. What is an Apex Email Service?
Apex Email Services allow developers to process incoming emails. The Apex class is used to define logic that extracts information from emails and performs actions based on the content.
Explanation: Email services in Apex enable automation by allowing applications to handle incoming email data programmatically.
32. What are Batchable Apex Interfaces?
Batchable Apex Interfaces allow developers to process large sets of records asynchronously. By implementing the Database.Batchable
interface, you can create custom batch jobs to process records in chunks.
Explanation: Batchable Apex is necessary for managing and processing large datasets efficiently while complying with governor limits.
33. What is the purpose of Governor Limits?
Governor Limits are put in place to ensure that no single tenant (organization) monopolizes resources in the shared Salesforce environment. They enforce limits on CPU time, queries, memory, and DML operations.
Explanation: Governor Limits ensure fair usage of Salesforce’s shared resources and prevent inefficient code from degrading system performance.
34. How do you bulkify your Apex code?
Bulkifying Apex code means making it capable of handling large data volumes by processing multiple records simultaneously, rather than one at a time. This is essential to avoid hitting governor limits.
Explanation: Bulkifying Apex code ensures that your application can handle large datasets without running into performance issues or hitting governor limits.
35. What is a Wrapper Class?
A Wrapper Class is a custom object that contains different types of data or objects. It is used to combine multiple data elements into a single object, often for display purposes.
Explanation: Wrapper Classes simplify the management of complex data structures, particularly in Visualforce pages or Lightning components.
36. What are Visualforce Controllers?
Visualforce Controllers are Apex classes that provide the logic behind a Visualforce page. They allow developers to retrieve data from Salesforce and manipulate it for display purposes.
Explanation: Visualforce Controllers provide a powerful way to add custom logic and data interactions to Salesforce Visualforce pages.
37. What is a Trigger Context Variable?
Trigger Context Variables are special variables in Apex triggers that contain information about the state of the records being processed. Examples include Trigger.new
, Trigger.old
, and Trigger.isInsert
.
Explanation: Understanding Trigger Context Variables is essential for writing efficient and accurate triggers in Apex.
Conclusion:
Salesforce Apex is a powerful programming language that allows developers to extend the capabilities of Salesforce through custom logic and automation. By mastering Apex, you can ensure efficient, reliable, and scalable applications that provide significant business value. Whether you’re new to Salesforce or preparing for an interview, understanding these core concepts will give you the foundation needed to succeed.
If you’re looking to take your career to the next level, be sure to check out our resume builder, where you can create a professional resume effortlessly. Also, explore our free resume templates and resume examples for more inspiration.
Recommended Reading: