SwiftUI, introduced by Apple in 2019, has quickly become a go-to framework for building UI for iOS, macOS, watchOS, and tvOS. With its declarative syntax and ease of use, SwiftUI simplifies the process of creating dynamic user interfaces across all Apple platforms. If you are preparing for an interview that involves SwiftUI, it’s crucial to be familiar with key concepts and questions that may come your way. In this article, we will cover the top 33 SwiftUI interview questions, providing concise answers and explanations to help you excel in your interview.
Top 33 SwiftUI Interview Questions
1. What is SwiftUI, and why is it used?
SwiftUI is Apple’s declarative framework for building user interfaces on all its platforms. It allows developers to design UIs by describing what they want, and SwiftUI handles the updates and rendering. It is used to create efficient and reusable UIs with less code and supports real-time previewing of design changes.
Explanation:
SwiftUI is favored because of its declarative nature, simplicity, and compatibility across Apple platforms, significantly reducing development time and errors.
2. How does SwiftUI differ from UIKit?
SwiftUI is a declarative framework, meaning developers state what the UI should look like, and the system automatically manages the UI’s state. UIKit, on the other hand, is imperative, requiring developers to manage the state and behavior of the UI manually.
Explanation:
SwiftUI abstracts much of the boilerplate code and complexities found in UIKit, making development faster and cleaner.
3. What is a @State
in SwiftUI?
@State
is a property wrapper in SwiftUI that allows you to manage and track changes to a view’s state. When a state changes, SwiftUI automatically re-renders the affected parts of the UI.
Explanation:
Using @State
helps keep your UI reactive and in sync with data changes without manual intervention.
4. Explain the role of @Binding
in SwiftUI.
@Binding
is a property wrapper that allows a child view to read and write a value owned by its parent view. It’s used for two-way data communication between views.
Explanation:@Binding
is essential for maintaining data consistency between views, promoting reusable components.
5. What are SwiftUI’s key advantages over other UI frameworks?
SwiftUI’s key advantages include a declarative syntax, real-time previews in Xcode, cross-platform compatibility, and reduced boilerplate code. These features enable faster development and easier maintenance.
Explanation:
SwiftUI’s simplicity and efficiency make it an ideal choice for Apple ecosystem development, streamlining the creation of responsive UIs.
Build your resume in just 5 minutes with AI.
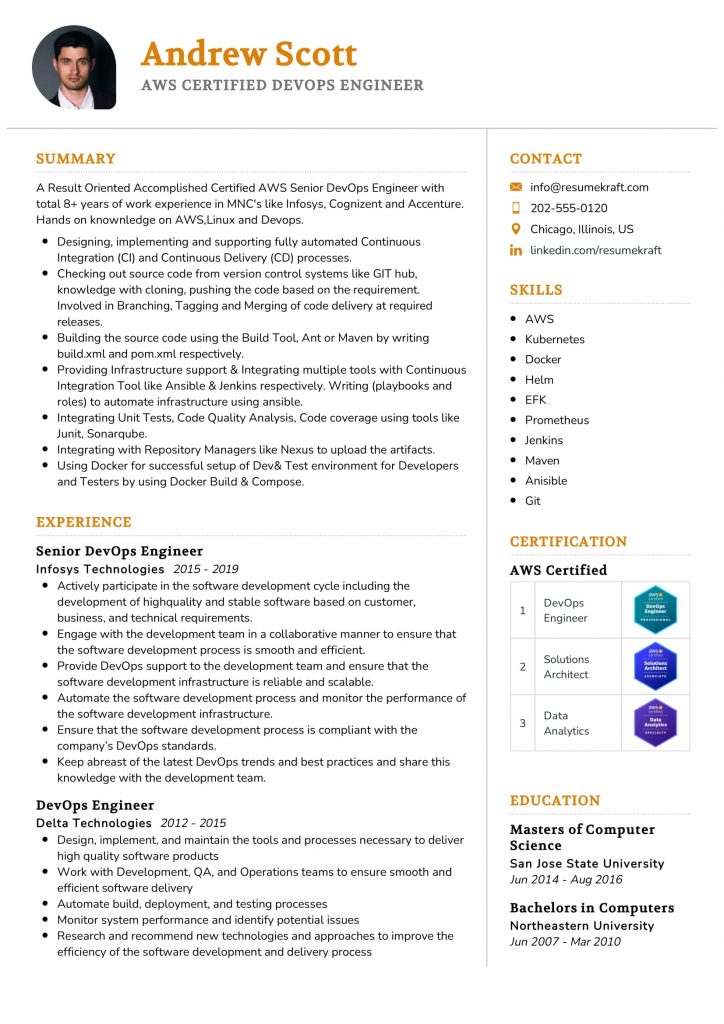
6. How does SwiftUI handle layout?
SwiftUI uses a system called “layout priority,” and views like HStack
, VStack
, and ZStack
to organize components. The framework calculates the optimal layout based on the constraints and available space.
Explanation:
SwiftUI’s layout engine automatically adjusts elements based on the space available, reducing the need for manual adjustments.
7. What is the purpose of @ObservedObject
in SwiftUI?
@ObservedObject
is used to observe and react to changes in external data models that conform to the ObservableObject
protocol. It helps SwiftUI re-render views when the model’s data changes.
Explanation:
This property wrapper is ideal for handling complex data models and ensuring that the UI stays in sync with data changes.
8. Explain @Environment
and its usage.
@Environment
is a property wrapper used to access values shared across different views, such as app-wide settings or theme preferences. It simplifies the process of passing data down the view hierarchy.
Explanation:@Environment
is essential for sharing global information between views without explicitly passing data through every layer.
9. What are the limitations of SwiftUI?
Some limitations of SwiftUI include lack of support for certain advanced features (compared to UIKit), steeper learning curve for experienced UIKit developers, and limitations in backward compatibility, especially with iOS versions prior to iOS 13.
Explanation:
Despite its simplicity, SwiftUI is still evolving, and some features might be harder to implement compared to UIKit.
10. What is a ViewBuilder
in SwiftUI?
A ViewBuilder
is a type of result builder that simplifies the process of building views in SwiftUI. It allows you to define multiple views in a declarative manner and returns the appropriate content.
Explanation:
It helps group multiple views together into a single return statement, making SwiftUI code more readable and concise.
11. How does ForEach
work in SwiftUI?
ForEach
is used to create a collection of views dynamically. It iterates over data collections like arrays and generates views for each item in the collection.
Explanation:
Using ForEach
is crucial for rendering lists or grids where the number of items is determined at runtime.
12. What are modifiers in SwiftUI?
Modifiers are methods that change or customize a view. SwiftUI provides a wide range of modifiers to alter a view’s appearance or behavior, such as .padding()
, .background()
, or .cornerRadius()
.
Explanation:
Modifiers offer a straightforward way to customize views without creating new components, enhancing code readability.
13. What is the difference between Spacer
and Divider
?
Spacer
is used to add flexible space between views in a stack, whereas Divider
is used to draw a visual line between views.
Explanation:
While both help in layout management, Spacer
ensures visual balance, and Divider
improves UI separation.
14. What is ZStack
used for in SwiftUI?
ZStack
overlays multiple views on top of each other. It allows you to stack views along the z-axis, which is useful for creating layered effects in your UI.
Explanation:ZStack
is commonly used for designing UIs with overlapping elements, such as a text overlay on an image.
15. What is the role of the NavigationView
in SwiftUI?
NavigationView
is a container that enables navigation between views. It provides navigation bars and allows users to push or pop views from the stack.
Explanation:NavigationView
is essential for apps that require hierarchical navigation, offering a smooth and consistent user experience.
16. Explain the concept of GeometryReader
in SwiftUI.
GeometryReader
is a view that gives access to the size and position of the parent container. It is used to dynamically adjust layouts based on the available space.
Explanation:
This view is crucial for building responsive UIs, as it provides data on container dimensions for custom layouts.
17. How do animations work in SwiftUI?
SwiftUI offers built-in support for animations using modifiers like .animation()
, .transition()
, and .withAnimation()
. You can animate changes in state and view properties seamlessly.
Explanation:
Animations in SwiftUI are declarative, making it easier to add smooth transitions and visual effects to your app.
18. What is a List
in SwiftUI, and how is it different from ForEach
?
List
is a scrollable container used to display rows of data. While ForEach
generates a collection of views, List
provides additional features like built-in scrolling and row management.
Explanation:List
is ideal for creating scrollable views, while ForEach
is more flexible for rendering non-scrollable data collections.
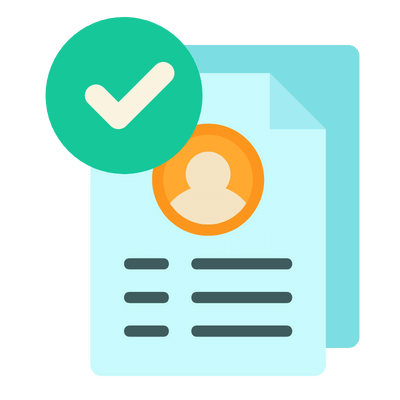
Build your resume in 5 minutes
Our resume builder is easy to use and will help you create a resume that is ATS-friendly and will stand out from the crowd.
19. Explain how PreferenceKey
is used in SwiftUI.
PreferenceKey
is a mechanism to propagate values up the view hierarchy in SwiftUI. It’s used when child views need to pass data back to their parent views.
Explanation:PreferenceKey
provides a way to transfer data between distant views, making it helpful for complex UIs.
20. What is the use of the Alert
view in SwiftUI?
Alert
is a pop-up dialog used to inform the user of important information or ask for confirmation. It can display multiple buttons for different user responses.
Explanation:
Alerts are vital for enhancing user interaction and ensuring they make informed choices during critical app moments.
21. How do you implement dark mode in SwiftUI?
SwiftUI automatically adapts to the system-wide dark mode settings. You can customize your app’s dark mode appearance using the .colorScheme()
modifier or by defining custom colors that adapt to light and dark modes.
Explanation:
Supporting dark mode improves user experience and aligns your app with modern system aesthetics.
22. How do you handle user input in SwiftUI?
In SwiftUI, you can handle user input through views like TextField
, TextEditor
, and Slider
, combined with @State
or @Binding
to manage input values.
Explanation:
SwiftUI’s data binding system makes it easy to capture and react to user input without much manual effort.
23. What is the purpose of @Published
in SwiftUI?
@Published
is used inside an ObservableObject
class to mark properties that trigger UI updates when changed. SwiftUI listens for changes to these properties and automatically updates the UI.
Explanation:
This is useful for managing dynamic content and ensuring the UI stays in sync with model data.
24. How do you display lists in SwiftUI?
Lists
in SwiftUI are created using the List
view. You can populate the list with dynamic data using ForEach
within the List
, or use static views for each row.
Explanation:
SwiftUI’s List
simplifies rendering scrollable data collections, similar to UITableView
in UIKit.
25. What are SwiftUI’s system requirements?
SwiftUI is available on iOS 13, macOS 10.15, watchOS 6, and tvOS 13 and later. It requires Xcode 11 or newer for development.
Explanation:
SwiftUI’s system requirements ensure compatibility with Apple’s latest platforms and features.
26. How does @MainActor
work in SwiftUI?
@MainActor
is used to ensure that certain pieces of code run on the main thread, which is crucial for updating the UI in SwiftUI since UI updates must occur on the main thread.
Explanation:@MainActor
is essential for maintaining thread safety when working with UI components.
27. What is Scene
in SwiftUI?
Scene
is the starting point for a SwiftUI app and defines the window or container where the app’s content will be displayed. It is used in SwiftUI’s App lifecycle model.
Explanation:
In the SwiftUI App lifecycle, Scene
defines the core structure of the app and how its views are organized.
28. How do you manage multiple windows in SwiftUI?
In macOS or iPadOS, you can manage multiple windows in SwiftUI by using WindowGroup
. Each window can contain different views or duplicate the same content.
Explanation:
Multiple window management improves multitasking and enhances productivity on larger devices like iPads or Macs.
29. How does SwiftUI handle accessibility?
SwiftUI provides built-in accessibility support with modifiers like .accessibilityLabel()
, .accessibilityValue()
, and .accessibilityHint()
. This ensures your app is usable by people with disabilities.
Explanation:
SwiftUI makes it easy to build accessible apps by integrating accessibility features directly into the UI.
30. What is the difference between LazyVStack
and VStack
?
LazyVStack
renders views lazily, meaning it only creates views as they are needed. VStack
, on the other hand, creates all its child views upfront.
Explanation:
Using LazyVStack
improves performance in scrollable containers by rendering only the visible views.
31. How do you create a button with an action in SwiftUI?
You create a button in SwiftUI using the Button
view, specifying the label and action closure. When tapped, the action is triggered.
Explanation:
Buttons in SwiftUI provide an intuitive way for users to interact with the app, triggering functions when pressed.
32. What is NavigationLink
in SwiftUI?
NavigationLink
is used for navigating between views within a NavigationView
. It creates a link that, when tapped, pushes the destination view onto the navigation stack.
Explanation:NavigationLink
is essential for implementing navigation patterns within hierarchical or multi-screen apps.
33. How do you present a modal in SwiftUI?
Modals in SwiftUI are presented using the .sheet()
modifier. This displays a view in a modal fashion, allowing the user to dismiss it when done.
Explanation:
Presenting modals is a common pattern for showing temporary or additional content without navigating away from the main view.
Conclusion
Preparing for a SwiftUI interview involves mastering both foundational and advanced concepts within the framework. The questions and answers provided here should give you a well-rounded understanding of what to expect. With SwiftUI’s increasing adoption, being well-versed in its features is essential for developers. Whether you are aiming for a position in iOS, macOS, or cross-platform development, the insights from this article will help you feel more confident in tackling technical interviews.
For more guidance on advancing your career, check out our resume builder to create a standout resume, or explore our free resume templates and resume examples to craft the perfect job application. Best of luck with your SwiftUI interview preparation!
Recommended Reading: